8. Additional PPIs¶
8.1. Introduction¶
Architectural PPIs described a collection of architecturally required PPIs. These were interfaces consumed by the PEI Foundation and are not intended to be consumed by other PEIMs.
In addition to these architectural PPIs, however, there is another name space of PPIs that are optional or mandatory for a given platform. This section describes these additional PPIs:
Required PPIs:
CPU I/O PPI
PCI Configuration PPI
Stall PPI
PEI Variable Services
Optional PPIs:
Security (SEC) Platform Information PPI
These shall be referred to as first-class PEIMs in some contexts.
8.2. Required Additional PPIs¶
8.2.1. PCI Configuration PPI (Required)¶
The PEI phase provides limited support for initializing and configuring PCI devices through the EFI_PEI_PCI_CFG2_PPI. The PEI module which supports a PCI root bridge may install this PPI to allow access to the PCI configuration space for a particular PCI segment. The PEI module responsible for the PCI root bridge representing segment 0 should also install a pointer to the PPI in the PEI Services Table.
The PEI modules which control devices on segment 0 may use the pointer provided in the PEI Services Table. The PEI modules for devices residing on other segments may find the correct PPI by iterating through PPI instances using the LocatePpi() function. For example:
EFI_STATUS Status;
UINTN Instance = 0;
EFI_PEI_PPI_DESCRIPTOR *PciCfgDescriptor = NULL;
EFI_PEI_PCI_CFG2_PPI *PciCfg = NULL;
/* Loop through all instances of the PPI */
for (;;) {
Status = PeiServices->LocatePpi(PeiServices,
&gPeiPciCfg2PpiGuid,
Instance,
&PciCfgDescriptor,
(VOID**) &PciCfg
);
if (Status != EFI_SUCCESS \|\|
PciCfg->Segment == MySegment) {
break;
}
Instance++;
}
if (Status == EFI_SUCCESS) {
...PciCfg contains pointer...
}
8.2.1.1. EFI_PEI_PCI_CFG2_PPI¶
Summary
Provides platform or chipset-specific access to the PCI configuration space for a specific PCI segment.
GUID
static const EFI_GUID EFI_PEI_PCI_CFG2_PPI_GUID = \
{ 0x57a449a, 0x1fdc, 0x4c06, \
{ 0xbf, 0xc9, 0xf5, 0x3f, 0x6a, 0x99, 0xbb, 0x92 }}
Prototype
typedef struct \_EFI_PEI_PCI_CFG2_PPI {
EFI_PEI_PCI_CFG2_PPI_IO Read;
EFI_PEI_PCI_CFG2_PPI_IO Write;
EFI_PEI_PCI_CFG2_PPI_RW Modify;
UINT16 Segment;
} EFI_PEI_PCI_CFG2_PPI
Parameters
Read
PCI read services. See the
Read()
function description.
Write
PCI write services. See the
Write()
function description.
Modify
PCI read-modify-write services. See the
Modify()
function description.
Segment
The PCI bus segment which the specified functions will access.
Description
The EFI_PEI_PCI_CFG2_PPI
interfaces are used to abstract
accesses to the configuration space of PCI controllers
behind a PCI root bridge controller. There can be multiple
instances of this PPI in the system, one for each segment.
The pointer to the instance which describes segment 0 is
installed in the PEI Services Table.
The assignment of segment numbers is implementation specific.
The Modify()
service allows for space-efficient
implementation of the following common operations:
Reading a register
Changing some bit fields within the register
Writing the register value back into the hardware
The Modify()
service is a composite of the Read()
and
Write()
services.
Parameters
Register
Register number in PCI configuration space.
Function
Function number in the PCI device (0-7).
Device
Device number in the PCI device (0-31).
Bus
PCI bus number (0-255).
ExtendedRegister
Register number in PCI configuration space. If this field is zero, then Register is used for the register number. If this field is non-zero, then Register is ignored and this field is used for the register number.
8.2.1.2. EFI_PEI_PCI_CFG2_PPI.Read()¶
Summary
PCI read operation.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_PCI_CFG_PPI_IO) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_PCI_CFG2_PPI *This,
IN EFI_PEI_PCI_CFG_PPI_WIDTH Width,
IN UINT64 Address,
IN OUT VOID *Buffer
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Width
The width of the access. Enumerated in bytes. Type
EFI_PEI_PCI_CFG_PPI_WIDTH
is defined in “Related Definitions” below.
Address
The physical address of the access. The format of the address is described by
EFI_PEI_PCI_CFG_PPI_PCI_ADDRESS
, which is defined in “Related Definitions” below.
Buffer
A pointer to the buffer of data.
Description
The Read()
function reads from a given location in the PCI
configuration space.
//************************************************************
// EFI_PEI_PCI_CFG_PPI_WIDTH
//************************************************************
typedef enum {
EfiPeiPciCfgWidthUint8 = 0,
EfiPeiPciCfgWidthUint16 = 1,
EfiPeiPciCfgWidthUint32 = 2,
EfiPeiPciCfgWidthUint64 = 3,
EfiPeiPciCfgWidthMaximum
} EFI_PEI_PCI_CFG_PPI_WIDTH;
//************************************************************
// EFI_PEI_PCI_CFG_PPI_PCI_ADDRESS
//************************************************************
typedef struct {
UINT8 Register;
UINT8 Function;
UINT8 Device;
UINT8 Bus;
UINT32 ExtendedRegister;
} EFI_PEI_PCI_CFG_PPI_PCI_ADDRESS;
Register
8-bit register offset within the PCI configuration space for a given device’s function space.
Function
Only the 3 least-significant bits are used to encode one of 8 possible functions within a given device.
Device
Only the 5 least-significant bits are used to encode one of 32 possible devices.
Bus
8-bit value to encode between 0 and 255 buses.
ExtendedRegister
Register number in PCI configuration space. If this field is zero, then Register is used for the register number. If this field is non-zero, then Register is ignored and this field is used for the register number.
#define EFI_PEI_PCI_CFG_ADDRESS(bus,dev,func,reg) \
(((bus) << 24) | \
((dev) << 16) | \
((func) << 8) | \
((reg) < 256 ? (reg) : ((UINT64) (reg) << 32)))
Status Codes Returned
EFI_SUCCESS |
The function completed successfully |
EFI_DEVICE_ERROR |
There was a problem with the transaction |
EFI_DEVICE_NOT_READY |
The device is not capable of supporting the operation at this time |
8.2.1.3. EFI_PEI_PCI_CFG2_PPI.Write()¶
Summary
PCI write operation.
Prototype
typedef
EFI_STATUS
(EFIAPI \*EFI_PEI_PCI_CFG_PPI_IO) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_PCI_CFG2_PPI *This,
IN EFI_PEI_PCI_CFG_PPI_WIDTH Width,
IN UINT64 Address,
IN OUT VOID *Buffer
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Width
The width of the access. Enumerated in bytes. Type
EFI_PEI_PCI_CFG_PPI_WIDTH
is defined inRead()
.
Address
The physical address of the access.
Buffer
A pointer to the buffer of data.
Description
The Write()
function writes to a given location in the PCI
configuration space.
Status Codes Returned
EFI_SUCCESS |
The function completed successfully |
EFI_DEVICE_ERROR |
There was a problem with the transaction |
EFI_DEVICE_NOT_READY |
The device is not capable of supporting the operation at this time |
8.2.1.4. EFI_PEI_PCI_CFG2_PPI.Modify()¶
Summary
PCI read-modify-write Operation.
Prototype
typedef
EFI_STATUS
(EFIAPI \*EFI_PEI_PCI_CFG_PPI_RW) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_PCI_CFG_PPI *This,
IN EFI_PEI_PCI_CFG_PPI_WIDTH Width,
IN UINT64 Address,
IN VOID *SetBits,
IN VOID *ClearBits
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Width
The width of the access. Enumerated in bytes. Type
EFI_PEI_PCI_CFG_PPI_WIDTH
is defined inRead()
.
Address
The physical address of the access.
SetBits
Points to value to bitwise-OR with the read configuration value. The size of the value is determined by Width.
ClearBits
Points to the value to negate and bitwise-AND with the read configuration value. The size of the value is determined by Width.
Description
The Modify()
function performs a read-modify-write
operation on the contents from a given location in the PCI
configuration space.
Status Codes Returned
EFI_SUCCESS |
The function completed successfully |
EFI_DEVICE_ERROR |
There was a problem with the transaction |
EFI_DEVICE_NOT_READY |
The device is not capable of supporting the operation at this time |
8.2.2. Stall PPI (Required)¶
8.2.2.1. EFI_PEI_STALL_PPI (Required)¶
Summary
This PPI is installed by some platform or chipset-specific PEIM that abstracts the blocking stall service to other agents.
GUID
#define EFI_PEI_STALL_PPI_GUID \
{ 0x1f4c6f90, 0xb06b, 0x48d8, {0xa2, 0x01, 0xba, 0xe5, \
0xf1, 0xcd, 0x7d, 0x56} }
PPI Interface Structure
typedef
struct _EFI_PEI_STALL_PPI {
UINTN Resolution;
EFI_PEI_STALL Stall;
} EFI_PEI_STALL_PPI;
Parameter
Resolution
The resolution in microseconds of the stall services.
Stall
The actual stall procedure call. See the
Stall()
function description.
Description
This service provides a simple, blocking stall with platform-specific resolution.
8.2.2.2. EFI_PEI_STALL_PPI.Stall()¶
Summary
Blocking stall.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_STALL) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_STALL_PPI *This,
IN UINTN Microseconds
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to the local data for the interface.
Microseconds
Number of microseconds for which to stall.
Description
The Stall()
function provides a blocking stall for at
least the number of microseconds stipulated in the final
argument of the API.
Status Codes Returned
EFI_SUCCESS |
The service provided at least the required delay. |
8.2.3. Variable Services PPI (Required)¶
8.2.3.1. EFI_PEI_READ_ONLY_VARIABLE2_PPI¶
Summary
Permits read-only access to the UEFI variable store during the PEI phase.
GUID
#define EFI_PEI_READ_ONLY_VARIABLE2_PPI_GUID \
{ 0x2ab86ef5, 0xecb5, 0x4134, \
0xb5, 0x56, 0x38, 0x54, 0xca, 0x1f, 0xe1, 0xb4 }
Prototype
typedef struct _EFI_PEI_READ_ONLY_VARIABLE2_PPI {
EFI_PEI_GET_VARIABLE2 GetVariable;
EFI_PEI_GET_NEXT_VARIABLE_NAME2 NextVariableName;
} EFI_PEI_READ_ONLY_VARIABLE2_PPI;
Parameters
GetVariable
A service to read the value of a particular variable using its name.
NextVariableName
Find the next variable name in the variable store.
Description
These services provide a light-weight, read-only variant of the full UEFI variable services.
8.2.3.2. EFI_PEI_READ_ONLY_VARIABLE2_PPI.GetVariable¶
Summary
This service retrieves a variable’s value using its name and GUID.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_GET_VARIABLE2)(
IN CONST EFI_PEI_READ_ONLY_VARIABLE2_PPI *This,
IN CONST CHAR16 *VariableName,
IN CONST EFI_GUID *VariableGuid,
OUT UINT32 *Attributes, OPTIONAL
IN OUT UINTN *DataSize,
OUT VOID *Data, OPTIONAL
);
Parameters
This
A pointer to this instance of the
EFI_PEI_READ_ONLY_VARIABLE2_PPI
.
VariableName
A pointer to a null-terminated string that is the variable’s name.
VariableGuid
A pointer to an
EFI_GUID
that is the variable’s GUID. The combination ofVariableGuid
andVariableName
must be unique.
Attributes
If non-NULL, on return, points to the variable’s attributes. See “Related Definitons” below for possible attribute values. If not NULL, then Attributes is set on output both when EFI_SUCCESS and when EFI_BUFFER_TOO_SMALL is returned.
DataSize
On entry, points to the size in bytes of the
Data
buffer. On return, points to the size of the data returned inData
.
Data
Points to the buffer which will hold the returned variable value. May be
NULL
with a zeroDataSize
in order to determine the size of the buffer needed.
Description
Read the specified variable from the UEFI variable store. If the Data buffer is too small to hold the contents of the variable, the error EFI_BUFFER_TOO_SMALL is returned and DataSize is set to the required buffer size to obtain the data.
Status Codes Returned
EFI_SUCCESS |
The variable was read successfully |
EFI_NOT_FOUND |
The variable was not found |
EFI_BUFFER_TOO_SMALL |
The |
EFI_INVALID_PARAMETER |
|
EFI_INVALID_PARAMETER |
|
EFI_INVALID_PARAMETER |
The |
EFI_DEVICE_ERROR |
The variable could not be retrieved because of a device error |
EFI_INVALID_PARAMETER |
|
8.2.3.3. EFI_PEI_READ_ONLY_VARIABLE2_PPI.NextVariableName¶
Summary
Return the next variable name and GUID.
Prototype
typedef
EFI_STATUS
(EFIAPI EFI_PEI_GET_NEXT_VARIABLE_NAME2) (
IN CONST EFI_PEI_READ_ONLY_VARIABLE2_PPI *This,
IN OUT UINTN *VariableNameSize,
IN OUT CHAR16 *VariableName,
IN OUT EFI_GUID *VariableGuid
);
Parameters
This
A pointer to this instance of the
EFI_PEI_READ_ONLY_VARIABLE2_PPI
.
VariableNameSize
On entry, points to the size of the buffer pointed to by
VariableName
. On return, the size of the variable name buffer
VariableName
On entry, a pointer to a null-terminated string that is the variable’s name. On return, points to the next variable’s null-terminated name string.
VariableGuid
On entry, a pointer to an
EFI_GUID
that is the variable’s GUID. On return, a pointer to the next variable’s GUID.
Desription
This function is called multiple times to retrieve the
VariableName
and VariableGuid
of all variables currently
available in the system. On each call, the previous results
are passed into the interface, and, on return, the interface
returns the data for the next interface. When the entire
variable list has been returned, EFI_NOT_FOUND
is
returned.
Note: If ``EFI_BUFFER_TOO_SMALL`` is returned, the ``VariableName`` buffer was too small for the name of the next variable. When such an error occurs, ``VariableNameSize`` is updated to reflect the size of the buffer needed. In all cases when calling ``GetNextVariableName()`` the ``VariableNameSize`` must not exceed the actual buffer size that was allocated for ``VariableName``.
To start the search, a null-terminated string is passed in
VariableName
; that is, VariableName
is a pointer to a
null Unicode character. This is always done on the initial
call. When VariableName
is a pointer to a null Unicode
character, VariableGuid
is ignored.
Status Codes Returned
EFI_SUCCESS |
The variable was read successfully |
EFI_NOT_FOUND |
The variable could not be found |
EFI_BUFFER_TOO_SMALL |
The |
EFI_INVALID_PARAMETER |
VariableName VariableGuid or VariableNameSize is |
EFI_DEVICE_ERROR |
The variable could not be retrieved because of a device error |
8.2.4. EFI DELAYED DISPATCH PPI (Required)¶
8.2.4.1. EFI_DELAYED_DISPATCH_PPI¶
Summary
Provide timed event service in PEI
GUID
#define EFI_DELAYED_DISPATCH_PPI_GUID \\
{ \
0x869c711d, 0x649c, 0x44fe, { 0x8b, 0x9e, 0x2c, 0xbb, 0x29,
0x11, 0xc3, 0xe6} } \
}
PPI Interface Structure
struct _EFI_DELAYED_DISPATCH_PPI {
EFI_DELAYED_DISPATCH_REGISTER Register;
EFI_DELAYED_DISPATCH WAIT_ON_EVENT WaitOnEvent;
};
Parameters
- Register
Register a callback to be called after a minimum delay has occurred.
- WaitOnEvent
Pause current process and allow other components to continue to dispatch until all entries with specified “DelayedGroupId” have completed.
Description
Define a time based event mechanism for PEI (Pei Delayed Dispatch):
Eliminate spin loops for timing. Instead of blocking for 50 or 100 ms, the driver would request callbacks after 50ms (or 100ms) and allow other PEIM’s to dispatch. Each loop through the PEI dispatcher will attempt to dispatch each of the queued delayed dispatch requests.
Method to schedule a callback after a minimum delay, not a real time callback. Maintains a 64 bit “State” for use by the callback.
PPI has two functions:
Register, to register a function to be called after the required delay with the current value of “State”.
WaitOnEvent
8.2.4.2. EFI_DELAYED_DISPATCH_PPI.Register()¶
Summary
Register a callback to be called after a minimum delay has occurred.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_DELAYED_DISPATCH_REGISTER)(
IN EFI_DELAYED_DISPATCH_PPI *This,
IN EFI_DELAYED_DISPATCH_FUNCTION Function,
IN UINT64 Context,
IN EFI_GUID *DelayedGroupId OPTIONAL,
IN UINT32 Delay
);
Parameters
- This
Pointer to the EFI_DELAYED_DISPATCH_PPI instance.
- Function
Function to call back. The function prototype is defined in the “Related Definitions” below.
- Context
Context data.
- DelayedGroupId
GUID for identifying the delayed dispatch request.
- Delay
Delay interval. This parameter describes a multiple of microseconds.
Description
This function is invoked by a PEIM to have a handler returned. The handler will be invoked after at the minimum of specified Delay. Note that if the Register
service is called with a Delay of zero, then the handler will be dispatched immediately. If the DelayedGroupId is specified, this request will be marked with GUID for WaitOnEvent
usage.
Related Definitions
//*******************************
// EFI_DELAYED_DISPATCH_FUNCTION
//*******************************
typedef
VOID
(EFIAPI *EFI_DELAYED_DISPATCH_FUNCTION)(
IN OUT UINT64 *Context,
OUT UINT32 *NewDelay
);
- Context
Pointer to Context Data. Can be updated by routine.
- NewDelay
The new delay in us. Leave at 0 to unregister this callback. Upon return, if NewDelay is 0, the function is unregistered. If NewDelay is not zero, this routine will be called again after the new delay period.
Status Codes Returned (EFI_DELAYED_DISPATCH_PPI.Register)
EFI_SUCCESS |
Function successfully invoked |
EFI_INVALID_PARAMETER |
One of the arguments is not supported |
EFI_OUT_OF_RESOURCES |
No more entries |
8.2.4.3. EFI_DELAYED_DISPATCH_PPI.WaitOnEvent()¶
Summary
Pause current process and allow other components to continue to dispatch until all entries with specified “DelayedGroupId” have completed.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_DELAYED_DISPATCH_WAIT ON EVENT)(
IN EFI_DELAYED_DISPATCH_PPI *This,
IN EFI_GUID DelayedGroupId
);
Parameters
- This
Pointer to the EFI_DELAYED_DISPATCH_PPI instance.
- DelayedGroupId
Delayed dispatch request ID the caller will wait on.
Description
This function is invoked by a PEIM to wait until all specified DelayedGroupId events have been dispatched. The other events will continue to dispatch while this process is being paused.
Status Codes Returned
EFI_SUCCESS |
Function successfully invoked |
EFI_INVALID_PARAMETER |
One of the arguments is not supported |
8.3. Optional Additional PPIs¶
8.3.1. SEC Platform Information PPI (Optional)¶
8.3.1.1. EFI_SEC_PLATFORM_INFORMATION_PPI (Optional)¶
Summary
This service is the platform information for the PEI Foundation.
GUID
#define EFI_SEC_PLATFORM_INFORMATION_GUID \
{0x6f8c2b35, 0xfef4, 0x448d, 0x82, 0x56, 0xe1, \
0x1b, 0x19, 0xd6, 0x10, 0x77}
Prototype
typedef struct \_EFI_SEC_PLATFORM_INFORMATION_PPI {
EFI_SEC_PLATFORM_INFORMATION PlatformInformation;
} EFI_SEC_PLATFORM_INFORMATION_PPI;
Parameters
PlatformInformation
Conveys state information out of the SEC phase into PEI. See the
PlatformInformation()
function description.
Description
This service abstracts platform-specific information.
8.3.1.2. EFI_SEC_PLATFORM_INFORMATION_PPI.PlatformInformation()¶
Summary
This service is the single member of the
EFI_SEC_PLATFORM_INFORMATION_PPI
that conveys state
information out of the Security (SEC) phase into PEI.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_SEC_PLATFORM_INFORMATION) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN OUT UINT64 *StructureSize,
OUT EFI_SEC_PLATFORM_INFORMATION_RECORD *PlatformInformationRecord
);
Parameters
PeiServices
Pointer to the PEI Services Table.
StructureSize
Pointer to the variable describing size of the input buffer.
PlatformInformationRecord
Pointer to the
EFI_SEC_PLATFORM_INFORMATION_RECORD
. TypeEFI_SEC_PLATFORM_INFORMATION_RECORD
is defined in “Related Definitions” below.
Description
This service is published by the SEC phase. The SEC phase
handoff has an optional EFI_PEI_PPI_DESCRIPTOR
list as its
final argument when control is passed from SEC into the PEI
Foundation. As such, if the platform supports the built-in
self test (BIST) on IA-32 Intel architecture or the PAL-A
handoff state for Itanium® architecture, this information
is encapsulated into the data structure abstracted by this
service. This information is collected for the boot-strap
processor (BSP) on IA-32, and for Itanium architecture, it
is available on all processors that execute the PEI
Foundation.
The motivation for this service is that a specific processor register contains this information for each microarchitecture, but the PEI CIS avoids using specific processor registers. Instead, the PEI CIS describes callable interfaces across which data is conveyed. As such, this processor state information that is collected at the reset of the machine is mapped into a common interface. The expectation is that a manageability agent, such as a platform PEIM that logs information for the platform, would use this interface to determine the viability of the BSP and possibly select an alternate BSP if there are significant errors.
//******************************************************
// EFI_SEC_PLATFORM_INFORMATION_RECORD
//******************************************************
typedef union {
IA32_HANDOFF_STATUS IA32HealthFlags;
X64_HANDOFF_STATUS x64HealthFlags;
ITANIUM_HANDOFF_STATUS ItaniumHealthFlags;
} EFI_SEC_PLATFORM_INFORMATION_RECORD;
HealthFlags
Contains information generated by microcode, or hardware, about the state of the processor upon reset. Type
EFI_HEALTH_FLAGS
is defined below.
//******************************************************
// EFI_HEALTH_FLAGS
//******************************************************
typedef union {
struct {
UINT32 Status : 2;
UINT32 Tested : 1;
UINT32 Reserved1 :13;
UINT32 VirtualMemoryUnavailable : 1;
UINT32 Ia32ExecutionUnavailable : 1;
UINT32 FloatingPointUnavailable : 1;
UINT32 MiscFeaturesUnavailable : 1;
UINT32 Reserved2 :12;
} Bits;
UINT32 Uint32;
} EFI_HEALTH_FLAGS;
..FIX THIS REF!!
IA-32 and X64 have the BIST. See Health Flag Bit Format
for more information on EFI_HEALTH_FLAGS
.
The following two structures are for IA32 and x64.
typedef EFI_HEALTH_FLAGS X64_HANDOFF_STATUS;
typedef EFI_HEALTH_FLAGS IA32_HANDOFF_STATUS;
There is no instance of an
EFI_SEC_PLATFORM_INFORMATION_RECORD
for the ARM PI
binding.
For Itanium, the structure is as follows:
For details, see the Itanium Software Developers Manual,
Volume 2, Rev 2.2, Document Number: 245318-005 (SwDevMan)
Section 11.2.2.1 “Definition of SALE_ENTRY
State
Parameter” as indicated below.
typedef struct {
UINT8 BootPhase; // SALE_ENTRY state : 3 = Recovery_Check
// and 0 = RESET or Normal_Boot phase.
// See 'function' in SwDevMan Fig 11-8 and
// Table 11-3.
UINT8 FWStatus; // Firmware status on entry to SALE.
// See 'Status' in SwDevMan Fig 11-8 and
// Table 11-4.
UINT16 Reserved1;
UINT32 Reserved2;
UINT16 ProcId; // Geographically significant unique
// processor ID assigned by PAL.
// See 'proc_id' in SwDevMan Fig 11-9
// and Table 11-5.
UINT16 Reserved3;
UINT8 IdMask; // See 'id_mask' in SwDevMan
// Fig 11-9 and Table 11-5.
UINT8 EidMask; // See 'eid_mask' in SwDevMan
// Fig 11-9 and Table 11-5
UINT16 Reserved4;
UINT64 PalCallAddress; // Address to make PAL calls.
UINT64 PalSpecialAddress; // If the entry state is
// RECOVERY_CHECK, this
// contains the PAL_RESET
// return address, and if entry
// state is RESET, this contains
// address for PAL_authentication
// call.
UINT64 SelfTestStatus; // GR35 from PALE_EXIT state,
// See 'Self Test State' in
// SwDevMan Fig 11-10 and
// Table 11-6.
UINT64 SelfTestControl; // GR37 from PALE_EXIT state:
// See 'Self Test Control' in
// SwDevMan Fig 11-11.
UINT64 MemoryBufferRequired; // See GR38 Reset Layout
// in SwDevMan Table 11-2.
} ITANIUM_HANDOFF_STATUS;
Consult the PALE_RESET
Exit State in Software
Development Manual for Itanium regarding an
interpretation of these fields.
Status Codes Returned
EFI_SUCCESS |
The data was successfully returned |
EFI_BUFFER_TOO_SMALL |
The buffer was too small The current buffer size needed to hold the record is returned in StructureSize |
8.3.2. SEC Platform Information 2 PPI (Optional)¶
8.3.2.1. EFI_SEC_PLATFORM_INFORMATION2_PPI (Optional)¶
Summary
This service is the primary handoff state into the PEI Foundation. The Security (SEC) component creates the early, transitory memory environment and also encapsulates knowledge of at least the location of the Boot Firmware Volume (BFV).
GUID
#define EFI_SEC_PLATFORM_INFORMATION2_GUID \
{0x9e9f374b, 0x8f16, 0x4230,
{ 0x98, 0x24, 0x58, 0x46, 0xee, 0x76, 0x6a, 0x97}};
Prototype
typedef struct _EFI_SEC_PLATFORM_INFORMATION2_PPI {
EFI_SEC_PLATFORM_INFORMATION2 PlatformInformation2;
} EFI_SEC_PLATFORM_INFORMATION2_PPI;
Parameters
PlatformInformation2
Conveys state information out of the SEC phase into PEI for many CPU’s. See the
PlatformInformation2()
function description.
Description
This service abstracts platform-specific information for
many CPU’s. It is the multi-processor equivalent of
PlatformInformation
for implementations that synchronize
some, if not all CPU’s in the SEC phase.
8.3.2.2. EFI_SEC_PLATFORM_INFORMATION2_PPI.PlatformInformation2()¶
Summary
This service is the single member of the
EFI_SEC_PLATFORM_INFORMATION2_PPI
that conveys state
information out of the Security (SEC) phase into PEI.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_SEC_PLATFORM_INFORMATION2) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN OUT UINT64 *StructureSize,
OUT EFI_SEC_PLATFORM_INFORMATION_RECORD2 *PlatformInformationRecord2
);
Parameters
PeiServices
Pointer to the PEI Services Table.
StructureSize
Pointer to the variable describing size of the input buffer.
PlatformInformationRecord2
Pointer to the
EFI_SEC_PLATFORM_INFORMATION_RECORD2
. TypeEFI_SEC_PLATFORM_INFORMATION_RECORD2
is defined in “Related Definitions” below.
Descriptions
This service is published by the SEC phase.
//******************************************************
// EFI_SEC_PLATFORM_INFORMATION_RECORD2
//******************************************************
typedef struct {
UINT32 CpuLocation;
EFI_SEC_PLATFORM_INFORMATION_RECORD InfoRecord;
} EFI_SEC_PLATFORM_INFORMATION_CPU;
typedef struct {
UINT32 NumberOfCpus.
EFI_SEC_PLATFORM_INFORMATION_CPU CpuInstance [1];
} EFI_SEC_PLATFORM_INFORMATION_RECORD2;
The CPU location would be the local API ID.
Status Codes Returned
EFI_SUCCESS |
The data was successfully returned |
EFI_BUFFER_TOO_SMALL |
The buffer was too small The current buffer size needed to hold the record is returned in |
8.3.3. Loaded Image PPI (Optional)¶
8.3.3.1. EFI_PEI_LOADED_IMAGE_PPI¶
Summary
Notifies other drivers of the PEIM being initialized by the PEI Dispatcher.
GUID
#define EFI_PEI_LOADED_IMAGE_PPI_GUID \
{ 0xc1fcd448, 0x6300, 0x4458, \
0xb8, 0x64, 0x28, 0xdf, 0x1, 0x53, 0x64, 0xbc }
Prototype
typedef struct _EFI_PEI_LOADED_IMAGE_PPI {
EFI_PHYSICAL_ADDRESS ImageAddress,
UINT64 ImageSize,
EFI_PEI_FILE_HANDLE FileHandle
} EFI_PEI_LOADED_IMAGE_PPI;
Parameters
ImageAddress
Address of the image at the address where it will be executed.
ImageSize
Size of the image as it will be executed.
FileHandle
File handle from which the image was loaded. Can be NULL, indicating the image was not loaded from a handle.
Description
This interface is installed by the PEI Dispatcher after the image has been loaded and after all security checks have been performed, to notify other PEIMs of the files which are being loaded.
Note: The same PEIM may be initialized twice.
8.3.4. SEC HOB PPI¶
8.3.4.1. EFI_SEC_HOB_DATA_PPI¶
Summary
This PPI allows the SEC code to install HOBs into the HOB list.
GUID
#define EFI_SEC_HOB_DATA_PPI_GUID \
{0x3ebdaf20, 0x6667, 0x40d8,\
{0xb4, 0xee, 0xf5, 0x99, 0x9a, 0xc1, 0xb7, 0x1f}};
Protocol Interface Structure
typedef struct _EFI_SEC_HOB_DATA_PPI {
EFI_SEC_HOB_DATA_GET GetHobs;
} EFI_SEC_HOB_DATA_PPI;
Parameters
GetHobs
Retrieves a list of HOBs to install into the PEI HOB list.
Description
This PPI provides a way for the SEC code to pass zero or more HOBs in a HOB list.
8.3.4.2. EFI_SEC_HOB_DATA_PPI.GetHobs()¶
Summary
Return a pointer to a buffer containing zero or more HOBs that will be installed into the PEI HOB List.
Prototype
EFI_STATUS
(EFIAPI *EFI_SEC_HOB_DATA_GET) (
IN CONST EFI_SEC_HOB_DATA_PPI *This,
OUT EFI_HOB_GENERIC_HEADER **HobList
);
Parameters
This
Pointer to this PPI structure.
HobList
A pointer to a returned pointer to zero or more HOBs. If no HOBs are to be returned, then the returned pointer is a pointer to a HOB of type
EFI_HOB_TYPE_END_OF_HOB_LIST
.
Description
This function returns a pointer to a pointer to zero or more
HOBs, terminated with a HOB of type
EI_HOB_TYPE_END_OF_HOB_LIST
.
Note: The HobList must not contain a
EFI_HOB_HANDOFF_INFO_TABLE
HOB (PHIT) HOB.
Note: The HOBs pointed to by HobList must be formed as described in section 4.5.2 of Volume 3, “HOB Construction Rules” including the requirement that the list start on an 8-byte boundary.
Status Codes
EFI_SUCCESS |
This function completed successfully. |
EFI_UNSUPPORTED |
No HOBS are available |
8.3.5. Recovery¶
This section contains the definitions of the PPIs that are required on platforms that support firmware recovery. The table below explains the organization of this section and lists the PPIs that are defined in this section.
Section |
Summary |
PPI Definition |
---|---|---|
Recovery Module PPI |
Describes the main Recovery Module PPI |
EFI_PEI _RECOVERY_MODULE_PPI |
Device Recovery Module PPI |
Describes the Device Recovery Module PPI |
EFI_PEI_DEVICE _RECOVERY_MODULE_PPI |
Device Recovery Block I O PPI |
Describes the Device Recovery Block I O PPI This section is device specific and addresses the most common form of recovery media block I O devices such as legacy floppy CD ROM or IDE devices |
EFI_PEI_R ECOVERY_BLOCK_IO_PPI |
This section also contains the definitions for additional data types and structures that are subordinate to the structures in which they are called. The following types or structures can be found in “Related Definitions” of the parent protocol or function definition:
EFI_PEI_BLOCK_IO_MEDIA
EFI_PEI_BLOCK_DEVICE_TYPE
EFI_PEI_LBA
8.3.5.1. Recovery Module PPI¶
8.3.5.1.1. EFI_PEI_RECOVERY_MODULE_PPI¶
Summary
Finds and loads the recovery files.
GUID
#define EFI_PEI_RECOVERY_MODULE_PPI_GUID \
{0xFB6D9542, 0x612D, 0x4f45, 0x87, 0x2F, 0x5C, \
0xFF, 0x52, 0xE9, 0x3D, 0xCF}
PPI Interface Structure
typedef struct _EFI_PEI_RECOVERY_MODULE_PPI {
EFI_PEI_LOAD_RECOVERY_CAPSULE LoadRecoveryCapsule;
} EFI_PEI_RECOVERY_MODULE_PPI;
Parameters
LoadRecoveryCapsule
Loads a DXE binary capsule into memory.
Description
This module has many roles and is responsible for the following:
Calling the driver recovery PPI
EFI_PEI_DEVICE_RECOVERY_MODULE_PPI.GetNumberRecoveryCapsules()
to determine if one or more DXE recovery entities exist.If no capsules exist, then performing appropriate error handling.
Allocating a buffer of
MaxRecoveryCapsuleSize
as determined byEFI_PEI_DEVICE_RECOVERY_MODULE_PPI
.GetRecoveryCapsuleInfo()
or larger.Determining the policy in which DXE recovery capsules are loaded.
Calling the driver recovery PPI
EFI_PEI_DEVICE_RECOVERY_MODULE_PPI.LoadRecoveryCapsule()
for capsule number x.If the load failed, performing appropriate error handling.
Performing security checks for a loaded DXE recovery capsule.
If the security checks failed, then logging the failure in a data HOB.
If the security checks failed, then determining the next
EFI_PEI_DEVICE_RECOVERY_MODULE_PPI.LoadRecoveryCapsule()
capsule number; otherwise, go to step 11.If more DXE recovery capsules exist, then go to step 5; otherwise, perform error handling.
Decomposing the capsule loaded by
EFI_PEI_DEVICE_RECOVERY_MODULE_PPI.LoadRecoveryCapsule()
into its components. It is assumed that the path parameters are redundant for recovery and Setup parameters are either redundant or canned.Invalidating all HOB entries for updateable firmware volume entries. This invalidation prevents possible errant drivers from being executed.
Updating the HOB table with the recovery DXE firmware volume information generated from the capsule decomposition.
Returning to the PEI Dispatcher.
8.3.5.1.2. EFI_PEI_RECOVERY_MODULE_PPI.LoadRecoveryCapsule()¶
Summary
Loads a DXE capsule from some media into memory and updates the HOB table with the DXE firmware volume information.
Prototype
typedef
EFI_STATUS
(EFIAPI \*EFI_PEI_LOAD_RECOVERY_CAPSULE) (
IN EFI_PEI_SERVICES \ **PeiServices,
IN struct \_EFI_PEI_RECOVERY_MODULE_PPI *This
);
Parameters
PeiServices
General-purpose services that are available to every PEIM. Type EFI_PEI_SERVICES is defined in EFI_PEI_SERVICES .
This
Indicates the
EFI_PEI_RECOVERY_MODULE_PPI
instance.Description
This function, by whatever mechanism, retrieves a DXE capsule from some device and loads it into memory. Note that the published interface is device neutral.
Status Codes Returned
EFI_SUCCESS |
The capsule was loaded correctly. |
EFI_DEVICE_ERROR |
A devive error occured |
EFI_NOT_FOUND |
A recovery DXE capsule cannot be found. |
8.3.5.2. Device Recovery Module PPI¶
8.3.5.2.1. EFI_PEI_DEVICE_RECOVERY_MODULE_PPI¶
Summary
Presents a standard interface to
EFI_PEI_RECOVERY_MODULE_PPI
, regardless of the underlying
device(s).
GUID
#define EFI_PEI_DEVICE_RECOVERY_MODULE_PPI_GUID \
{ 0x0DE2CE25, 0x446A, 0x45a7, 0xBF, 0xC9, 0x37, 0xDA, \
0x26, 0x34, 0x4B, 0x37}
PPI Interface Structure
typedef struct \_EFI_PEI_DEVICE_RECOVERY_MODULE_PPI {
EFI_PEI_DEVICE_GET_NUMBER_RECOVERY_CAPSULE GetNumberRecoveryCapsules;
EFI_PEI_DEVICE_GET_RECOVERY_CAPSULE_INFO GetRecoveryCapsuleInfo;
EFI_PEI_DEVICE_LOAD_RECOVERY_CAPSULE LoadRecoveryCapsule;
} EFI_PEI_DEVICE_RECOVERY_MODULE_PPI;
Parameters
GetNumberRecoveryCapsules
Returns the number of DXE capsules that were found. See the
GetNumberRecoveryCapsules()
function description.
GetRecoveryCapsuleInfo
Returns the capsule image type and the size of a given image. See the
GetRecoveryCapsuleInfo()
function description.
LoadRecoveryCapsule
Loads a DXE capsule into memory. See the
LoadRecoveryCapsule()
function description.
Description
The role of this module is to present a standard interface
to EFI_PEI_RECOVERY_MODULE_PPI
, regardless of the
underlying device(s). The interface does the following:
Reports the number of recovery DXE capsules that exist on the associated device(s)
Finds the requested firmware binary capsule
Loads that capsule into memory
A device can be either a group of devices, such as a block device, or an individual device. The module determines the internal search order, with capsule number 1 as the highest load priority and number N as the lowest priority.
8.3.5.2.2. EFI_PEI_DEVICE_RECOVERY_MODULE_PPI.GetNumberRecoveryCapsules()¶
Summary
Returns the number of DXE capsules residing on the device.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_DEVICE_GET_NUMBER_RECOVERY_CAPSULE) (
IN EFI_PEI_SERVICES **PeiServices,
IN struct _EFI_PEI_DEVICE_RECOVERY_MODULE_PPI *This,
OUT UINTN *NumberRecoveryCapsules
);
Parameters
PeiServices
General-purpose services that are available to every PEIM. Type
EFI_PEI_SERVICES
is defined in EFI_PEI_SERVICES .
This
Indicates the
EFI_PEI_DEVICE_RECOVERY_MODULE_PPI
instance.
NumberRecoveryCapsules
Pointer to a caller-allocated
UINTN
. On output,*NumberRecoveryCapsules
contains the number of recovery capsule images available for retrieval from this PEIM instance.
Description
This function, by whatever mechanism, searches for DXE capsules from the associated device and returns the number and maximum size in bytes of the capsules discovered. Entry 1 is assumed to be the highest load priority and entry N is assumed to be the lowest priority.
Status Codes Returned
EFI_SUCCESS |
One or more capsules were discovered. |
EFI_DEVICE_ERROR |
A device error has occurred. |
EFI_NOT_FOUND |
A recovery DXE capsule cannot be found. |
8.3.5.2.3. EFI_PEI_DEVICE_RECOVERY_MODULE_PPI.GetRecoveryCapsuleInfo()¶
Summary
Returns the size and type of the requested recovery capsule.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_DEVICE_GET_RECOVERY_CAPSULE_INFO) (
IN EFI_PEI_SERVICES **PeiServices,
IN struct _EFI_PEI_DEVICE_RECOVERY_MODULE_PPI *This,
IN UINTN CapsuleInstance,
OUT UINTN *Size,
OUT EFI_GUID *CapsuleType
);
Parameters
PeiServices
General-purpose services that are available to every PEIM. Type
EFI_PEI_SERVICES
is defined in EFI_PEI_SERVICES.
This
Indicates the
EFI_PEI_DEVICE_RECOVERY_MODULE_PPI
instance.
CapsuleInstance
Specifies for which capsule instance to retrieve the information. This parameter must be between one and the value returned by
GetNumberRecoveryCapsules()
inNumberRecoveryCapsules
.
Size
A pointer to a caller-allocated
UINTN
in which the size of the requested recovery module is returned.
CapsuleType
A pointer to a caller-allocated
EFI_GUID
in which the type of the requested recovery capsule is returned. The semantic meaning of the value returned is defined by the implementation. TypeEFI_GUID
is defined inInstallProtocolInterface()
in the EFI 1.10 Specification .
Description
This function returns the size and type of the capsule specified by CapsuleInstance.
Status Codes Returned
EFI_SUCCESS |
One or more capsules were discovered. |
EFI_DEVICE_ERROR |
A device error occurred. |
EFI_NOT_FOUND |
A recovery DXE capsule cannot be found. |
8.3.5.2.4. EFI_PEI_DEVICE_RECOVERY_MODULE_PPI.LoadRecoveryCapsule()¶
Summary
Loads a DXE capsule from some media into memory.
Prototype
typedef
EFI_STATUS
(EFIAPI \*EFI_PEI_DEVICE_LOAD_RECOVERY_CAPSULE) (
IN EFI_PEI_SERVICES **PeiServices,
IN struct \_EFI_PEI_DEVICE_RECOVERY_MODULE_PPI *This,
IN UINTN CapsuleInstance,
OUT VOID *Buffer
);
Parameters
PeiServices
General-purpose services that are available to every PEIM. Type
EFI_PEI_SERVICES
is defined in EFI_PEI_SERVICES.
This
Indicates the
EFI_PEI_DEVICE_RECOVERY_MODULE_PPI
instance.
CapsuleInstance
Specifies which capsule instance to retrieve.
Buffer
Specifies a caller-allocated buffer in which the requested recovery capsule will be returned.
Description
This function, by whatever mechanism, retrieves a DXE capsule from some device and loads it into memory. Note that the published interface is device neutral.
Status Codes Returned
EFI_SUCCESS |
The capsule was loaded correctly. |
EFI_DEVICE_ERROR |
A device error occurred. |
EFI_NOT_FOUND |
The requested recovery DXE capsule cannot be found. |
8.3.5.3. Device Recovery Block I/O PPI¶
The Recovery Module PPI and the Device Recovery Module PPI subsections earlier in Code Definitions are device neutral. This section is device specific and addresses the most common form of recovery media-block I/O devices such as legacy floppy, CD-ROM, or IDE devices.
The Recovery Block I/O PPI is used to access block devices. Because the Recovery Block I/O PPIs that are provided by the PEI ATAPI driver and PEI legacy floppy driver are the same, here we define a set of general PPIs for both drivers to use.
8.3.5.3.1. EFI_PEI_RECOVERY_BLOCK_IO_PPI¶
Summary
Provides the services required to access a block I/O device during PEI recovery boot mode.
GUID
#define EFI_PEI_RECOVERY_BLOCK_IO_PPI_GUID \
{ 0x695d8aa1, 0x42ee, 0x4c46, 0x80, 0x5c,0x6e, 0xa6, \
0xbc, 0xe7, 0x99, 0xe3 }
PPI Interface Structure
typedef struct _EFI_PEI_RECOVERY_BLOCK_IO_PPI {
EFI_PEI_GET_NUMBER_BLOCK_DEVICES GetNumberOfBlockDevices;
EFI_PEI_GET_DEVICE_MEDIA_INFORMATION GetBlockDeviceMediaInfo;
EFI_PEI_READ_BLOCKS ReadBlocks;
} EFI_PEI_RECOVERY_BLOCK_IO_PPI;
Parameters
GetNumberOfBlockDevices
Gets the number of block I/O devices that the specific block driver manages. See the
GetNumberOfBlockDevices()
function description.
GetBlockDeviceMediaInfo
Gets the specified media information. See the
GetBlockDeviceMediaInfo()
function description.
ReadBlocks
Reads the requested number of blocks from the specified block device. See the
ReadBlocks()
function description.
Description
This function provides the services that are required to access a block I/O device during PEI recovery boot mode.
8.3.5.3.2. EFI_PEI_RECOVERY_BLOCK_IO_PPI.GetNumberOfBlockDevices()¶
Summary
Gets the count of block I/O devices that one specific block driver detects.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_GET_NUMBER_BLOCK_DEVICES) (
IN EFI_PEI_SERVICES **PeiServices,
IN struct _EFI_PEI_RECOVERY_BLOCK_IO_PPI *This,
OUT UINTN *NumberBlockDevices
);
Parameters
PeiServices
General-purpose services that are available to every PEIM. Type
EFI_PEI_SERVICES
is defined in EFI_PEI_SERVICES.
This
Indicates the
EFI_PEI_RECOVERY_BLOCK_IO_PPI
instance.
NumberBlockDevices
The number of block I/O devices discovered.
Description
This function is used for getting the count of block I/O devices that one specific block driver detects. To the PEI ATAPI driver, it returns the number of all the detected ATAPI devices it detects during the enumeration process. To the PEI legacy floppy driver, it returns the number of all the legacy devices it finds during its enumeration process. If no device is detected, then the function will return zero.
Status Codes Returned
EFI_SUCCESS |
Operation performed successfully |
8.3.5.3.3. EFI_PEI_RECOVERY_BLOCK_IO_PPI.GetBlockDeviceMediaInfo()¶
Summary
Gets a block device’s media information.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_GET_DEVICE_MEDIA_INFORMATION) (
IN EFI_PEI_SERVICES **PeiServices,
IN struct \_EFI_PEI_RECOVERY_BLOCK_IO_PPI *This,
IN UINTN DeviceIndex,
OUT EFI_PEI_BLOCK_IO_MEDIA *MediaInfo
);
Parameters
PeiServices
General-purpose services that are available to every PEIM. Type
EFI_PEI_SERVICES
is defined in EFI_PEI_SERVICES.
This
Indicates the
EFI_PEI_RECOVERY_BLOCK_IO_PPI
instance.
DeviceIndex
Specifies the block device to which the function wants to talk. Because the driver that implements Block I/O PPIs will manage multiple block devices, the PPIs that want to talk to a single device must specify the device index that was assigned during the enumeration process. This index is a number from one to
NumberBlockDevices
.
MediaInfo
The media information of the specified block media. Type
EFI_PEI_BLOCK_IO_MEDIA
is defined in “Related Definitions” below. The caller is responsible for the ownership of this data structure.
Note: This structure describes an enumeration of possible block device types. This enumeration exists because no device paths are actually passed across interfaces that describe the type or class of hardware that is publishing the block I/O interface. This enumeration will allow for policy decisions in the Recovery PEIM, such as “Try to recover from legacy floppy first, LS-120 second, CD-ROM third.” If there are multiple partitions abstracted by a given device type, they should be reported in ascending order; this order also applies to nested partitions, such as legacy MBR, where the outermost partitions would have precedence in the reporting order. The same logic applies to systems such as IDE that have precedence relationships like “Master/Slave” or “Primary/Secondary”; the master device should be reported first, the slave second.
Description
This function will provide the caller with the specified block device’s media information. If the media changes, calling this function will update the media information accordingly.
//***************************************************
// EFI_PEI_BLOCK_IO_MEDIA
//***************************************************
typedef struct {
EFI_PEI_BLOCK_DEVICE_TYPE DeviceType;
BOOLEAN MediaPresent;
UINTN LastBlock;
UINTN BlockSize;
} PEI_BLOCK_IO_MEDIA;
DevType
The type of media device being referenced by DeviceIndex. Type
EFI_PEI_BLOCK_DEVICE_TYPE
is defined below.
MediaPresent
A flag that indicates if media is present. This flag is always set for nonremovable media devices.
LastBlock
The last logical block that the device supports.
BlockSize
The size of a logical block in bytes.
//**********************************************************\*
// EFI_PEI_BLOCK_DEVICE_TYPE
//**********************************************************\*
typedef enum {
LegacyFloppy = 0,
IdeCDROM = 1,
IdeLS120 = 2,
UsbMassStorage = 3,
SD = 4,
EMMC = 5,
UfsDevice = 6,
MaxDeviceType
} EFI_PEI_BLOCK_DEVICE_TYPE;
Status Codes Returned
EFI_SUCCESS |
Media information about the specified block device was obtained successfully |
EFI_DEVICE_ERROR |
Cannot get the media information due to a hardware error |
8.3.5.3.4. EFI_PEI_RECOVERY_BLOCK_IO_PPI.ReadBlocks()¶
Summary
Reads the requested number of blocks from the specified block device.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_READ_BLOCKS) (
IN EFI_PEI_SERVICES **PeiServices,
IN struct _EFI_PEI_RECOVERY_BLOCK_IO_PPI *This,
IN UINTN DeviceIndex,
IN EFI_PEI_LBA StartLBA,
IN UINTN BufferSize,
OUT VOID *Buffer
);
Parameters
PeiServices
General-purpose services that are available to every PEIM. Type
EFI_PEI_SERVICES
is defined in (EFI_PEI_SERVICES) .
This
Indicates the
EFI_PEI_RECOVERY_BLOCK_IO_PPI
instance.
DeviceIndex
Specifies the block device to which the function wants to talk. Because the driver that implements Block I/O PPIs will manage multiple block devices, the PPIs that want to talk to a single device must specify the device index that was assigned during the enumeration process. This index is a number from one to
NumberBlockDevices
.
StartLBA
The starting logical block address (LBA) to read from on the device. Type
EFI_PEI_LBA
is defined in “Related Definitions” below.
BufferSize
The size of the
Buffer
in bytes. This number must be a multiple of the intrinsic block size of the device.
Buffer
A pointer to the destination buffer for the data. The caller is responsible for the ownership of the buffer.
Description
The function reads the requested number of blocks from the
device. All the blocks are read, or an error is returned. If
there is no media in the device, the function returns
EFI_NO_MEDIA
.
//****************************************************\*
// EFI_PEI_LBA
//****************************************************\*
typedef UINT64 EFI_PEI_LBA;
EFI_PEI_LBA
is the UINT64
LBA number.
Status Codes Returned
EFI_SUCCESS |
The data was read correctly from the device |
EFI_DEVICE_ERROR |
The device reported an error while attempting to perform the read operation |
EFI_INVALID_PARAMETER |
The read request contains LBAs that are not valid or the buffer is not properly aligned |
EFI_NO_MEDIA |
There is no media in the device |
EFI_BAD_BUFFER_SIZE |
The |
8.3.6. EFI PEI Recovery Block IO2 PPI¶
8.3.6.1. EFI_PEI_RECOVERY_BLOCK_IO2_PPI¶
Summary
Provides the services required to access a block I/O device during PEI recovery boot mode.
GUID
#define EFI_PEI_RECOVERY_BLOCK_IO2_PPI_GUID \
{ 0x26cc0fad, 0xbeb3, 0x478a,\
{ 0x91, 0xb2, 0xc, 0x18, 0x8f, 0x72, 0x61, 0x98 } }
PPI Interface Structure
typedef struct \_EFI_PEI_RECOVERY_BLOCK_IO2_PPI {
UINT64 Revision;
EFI_PEI_GET_NUMBER_BLOCK_DEVICES2 GetNumberOfBlockDevices;
EFI_PEI_GET_DEVICE_MEDIA_INFORMATION2
GetBlockDeviceMediaInfo;
EFI_PEI_READ_BLOCKS2 ReadBlocks:
} EFI_PEI_RECOVERY_BLOCK_IO2_PPI;
Parameters
Revision
The revision to which the interface adheres. All future revisions must be backwards compatible.
GetNumberOfBlockDevices
Gets the number of block I/O devices that the specific block driver manages. See the
GetNumberOfBlockDevices()
function description.
GetBlockDeviceMediaInfo
Gets the specified media information. See the
GetBlockDeviceMediaInfo()
function description.
ReadBlocks
Reads the requested number of blocks from the specified block device. See the
ReadBlocks()
function description.
#define EFI_PEI_RECOVERY_BLOCK_IO2_PPI_REVISION 0x00010000
Description
This function provides the services that are required to access a block I/O device during PEI recovery boot mode.
8.3.6.2. EFI_PEI_RECOVERY_BLOCK_IO2_PPI.GetNumberOfBlockDevices()¶
Summary
Gets the count of block I/O devices that one specific block driver detects.
Prototype
typedef
EFI_STATUS
(EFIAPI \*EFI_PEI_GET_NUMBER_BLOCK_DEVICES2) (
IN EFI_PEI_SERVICES **PeiServices,
IN EFI_PEI_RECOVERY_BLOCK_IO2_PPI *This,
OUT UINTN *NumberBlockDevices
);
Parameters
PeiServices
General-purpose services that are available to every PEIM. Type
EFI_PEI_SERVICES
is defined in the Intel*® *Platform Innovation Framework for EFI Pre-EFI Initialization Core Interface Specification (PEI CIS).
This
Indicates the
EFI_PEI_RECOVERY_BLOCK_IO_PPI
instance.
NumberBlockDevices
The number of block I/O devices discovered.
Description
This function is used for getting the count of block I/O devices that one specific block driver detects. To the PEI ATAPI driver, it returns the number of all the detected ATAPI devices it detects during the enumeration process. To the PEI legacy floppy driver, it returns the number of all the legacy devices it finds during its enumeration process. If no device is detected, then the function will return zero.
Status Codes Returned
EFI_SUCCESS |
Operation performed successfully |
8.3.6.3. EFI_PEI_RECOVERY_BLOCK_IO2_PPI.GetBlockDeviceMediaInfo()¶
Summary
Gets a block device’s media information.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_GET_DEVICE_MEDIA_INFORMATION2) (
IN EFI_PEI_SERVICES **PeiServices,
IN EFI_PEI_RECOVERY_BLOCK_IO2_PPI *This,
IN UINTN DeviceIndex,
OUT EFI_PEI_BLOCK_IO2_MEDIA *MediaInfo
);
Parameters
PeiServices
General-purpose services that are available to every PEIM. Type
EFI_PEI_SERVICES
is defined in the Intel*® *Platform Innovation Framework for EFI Pre-EFI Initialization Core Interface Specification (PEI CIS).
This
Indicates the
EFI_PEI_RECOVERY_BLOCK_IO_PPI
instance.
DeviceIndex
Specifies the block device to which the function wants to talk. Because the driver that implements Block I/O PPIs will manage multiple block devices, the PPIs that want to talk to a single device must specify the device index that was assigned during the enumeration process. This index is a number from one to
NumberBlockDevices
.
MediaInfo
The media information of the specified block media. Type
EFI_PEI_BLOCK_IO2_MEDIA
is defined in “Related Definitions” below. The caller is responsible for the ownership of this data structure.Note that this structure describes an enumeration of possible block device types. This enumeration exists because no device paths are actually passed across interfaces that describe the type or class of hardware that is publishing the block I/O interface. This enumeration will allow for policy decisions in the Recovery PEIM, such as “Try to recover from legacy floppy first, USB mass storage device second, CD-ROM third.” If there are multiple partitions abstracted by a given device type, they should be reported in ascending order; this order also applies to nested partitions, such as legacy MBR, where the outermost partitions would have precedence in the reporting order. The same logic applies to systems such as IDE that have precedence relationships like “Master/Slave” or “Primary/Secondary”; the master device should be reported first, the slave second.
Description
This function will provide the caller with the specified block device’s media information. If the media changes, calling this function will update the media information accordingly.
//**************************************************
// EFI_PEI_BLOCK_IO2_MEDIA
//**************************************************
typedef struct {
UINT8 InterfaceType;
BOOLEAN RemovablaMedia;
BOOLEAN MediaPresent;
BOOLEAN ReadOnly;
UINT32 BlockSize;
EFI_PEI_LBA LastBlock;
} PEI_BLOCK_IO2_MEDIA;
InterfaceType
A type of interface that the device being referenced by
DeviceIndex
is attached to. This field re-uses Messaging Device Path Node sub-type values as defined by Section “9.3.5 Messaging Device Path” of UEFI Specification . When more than one sub-type is associated with the interface, sub-type with the smallest number must be used. For example,InterfaceType
must be set to 5 for USB devices.
RemovablaMedia
A flag that indicates if media is removable.
MediaPresent
A flag that indicates if media is present. This flag is always set for non-removable media devices.
ReadOnly
A flag that indicates if media is read-only.
LastBlock
The last logical block that the device supports.
BlockSize
The size of a logical block in bytes. Type
EFI_PEI_LBA
is defined below.
//*****************************************************
// EFI_PEI_LBA
//*****************************************************
typedef UINT64 EFI_PEI_LBA;
EFI_PEI_LBA
is the UINT64
LBA number.
Status Codes Returned
EFI_SUCCESS |
Media information about the specified block device was obtained successfully |
EFI_DEVICE_ERROR |
Cannot get the media information due to a hardware error |
8.3.6.4. EFI_PEI_RECOVERY_BLOCK_IO2_PPI.ReadBlocks()¶
Summary
Reads the requested number of blocks from the specified block device.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_READ_BLOCKS2) (
IN EFI_PEI_SERVICES **PeiServices,
IN EFI_PEI_RECOVERY_BLOCK_IO2_PPI *This,
IN UINTN DeviceIndex,
IN EFI_PEI_LBA StartLBA,
IN UINTN BufferSize,
OUT VOID *Buffer
);
Parameters
PeiServices
General-purpose services that are available to every PEIM. Type
EFI_PEI_SERVICES
is defined in the Intel*® *Platform Innovation Framework for EFI Pre-EFI Initialization Core Interface Specification (PEI CIS).
This
Indicates the
EFI_PEI_RECOVERY_BLOCK_IO_PPI
instance.
DeviceIndex
Specifies the block device to which the function wants to talk. Because the driver that implements Block I/O PPIs will manage multiple block devices, the PPIs that want to talk to a single device must specify the device index that was assigned during the enumeration process. This index is a number from one to
NumberBlockDevices
.
StartLBA
The starting logical block address (LBA) to read from on the device. Type
EFI_PEI_LBA
is defined in in theGetBlockDeviceMediaInfo()
function description.
BufferSize
The size of the
Buffer
in bytes. This number must be a multiple of the intrinsic block size of the device.
Buffer
A pointer to the destination buffer for the data. The caller is responsible for the ownership of the buffer.
Description
The function reads the requested number of blocks from the
device. All the blocks are read, or an error is returned. If
there is no media in the device, the function returns
EFI_NO_MEDIA
.
Status Codes Returned
EFI_SUCCESS |
The data was read correctly from the device |
EFI_DEVICE_ERROR |
The device reported an error while attempting to perform the read operation |
EFI_INVALID_PARAMETER |
The read request contains LBAs that are not valid or the buffer is not properly aligned |
EFI_NO_MEDIA |
There is no media in the device |
EFI_BAD_BUFFER_SIZE |
The BufferSize parameter is not a multiple of the intrinsic block size of the device |
8.3.7. EFI PEI Vector Handoff Info PPI¶
8.3.7.1. EFI_PEI_VECTOR_HANDOFF_INFO_PPI (Optional)¶
Summary
The PPI that describes an array of interrupt and/or exception vectors that are in use and need to persist.
GUID
#define EFI_PEI_VECTOR_HANDOFF_INFO_PPI_GUID \
{ 0x3cd652b4, 0x6d33, 0x4dce, \
{ 0x89, 0xdb, 0x83, 0xdf, 0x97, 0x66, 0xfc, 0xca } }
Protocol Interface Structure
typedef struct _EFI_PEI_VECTOR_HANDOFF_INFO_PPI {
EFI_VECTOR_HANDOFF_INFO *Info;
} EFI_PEI_VECTOR_HANDOFF_INFO_PPI;
Parameters
Info
Pointer to an array of interrupt and /or exception vectors.
Description
This is an optional PPI that may be produced by SEC. If
present, it provides a description of the interrupt and/or
exception vectors that were established in the SEC Phase and
need to persist into PEI and DXE. This PPI is an array of
entries that is terminated by an entry whose Attribute
is
set to EFI_VECTOR_HANDOFF_LAST_ENTRY
.
If Attribute
is set to EFI_VECTOR_HANDOFF_DO_NOT_HOOK
,
then the associated handler for VectorNumber
must be
preserved in PEI and DXE.
If Attribute
is set to EFI_VECTOR_HANDOFF_HOOK_BEFORE
,
then VectorNumber may be used in PEI and DXE, but new
handlers must be invoked prior to when the existing handler
is called.
If Attribute
is set to EFI_VECTOR_HANDOFF_HOOK_AFTER
,
then the associated VectorNumber
may be used in PEI and
DXE, but new handlers must be called after the existing
handler is called.
EFI_PEI_VECTOR_HANDOFF_INFO_PPI_GUID
can also be used in
the PEI Phase to build a GUIDed HOB that contains an array
of EFI_VECTOR_HANDOFF_INFO
entries that describes the
interrupt and/or exception vectors in use in the PEI Phase.
This may be identical to the array passed up from SEC, or it
could be an array that is augmented with additional vectors
used in PEI Phase.
//
// System configuration table entry that points to the table
// in case an entity in DXE wishes to update/change the vector
// table contents.
//
// The table shall be stored in memory of type
// EfiBootServicesData.
//
#define EFI_VECTOR_HANDOFF_TABLE_GUID \
{0x996ec11c, 0x5397, 0x4e73, \
{0xb5, 0x8f, 0x82, 0x7e, 0x52, 0x90, 0x6d, 0xef}}
typedef struct {
UINT32 VectorNumber;
UINT32 Attribute;
EFI_GUID Owner;
} EFI_VECTOR_HANDOFF_INFO;
Parameters
VectorNumber
The interrupt or exception vector that is in use and must be preserved.
Attribute
A bitmask that describes the attributes of the interrupt or exception vector.
Owner
The GUID identifies the party who created the entry. For the
EFI_VECTOR_HANDOFF_DO_NOT_HOOK
case, this establishes the single owner.
8.3.8. CPU I/O PPI (Optional)¶
8.3.8.1. EFI_PEI_CPU_IO_PPI (Optional)¶
If the service is not available, the PEI Core service
EFI_PEI_CPU_IO_PPI
*CpuIo
member functions will have a
dummy function that return EFI_NOT_AVAILABLE_YET
;
Summary
This PPI is installed by some platform or chipset-specific PEIM that abstracts the processor-visible I/O operations.
GUID
#define EFI_PEI_CPU_IO_PPI_INSTALLED_GUID \
{0xe6af1f7b, 0xfc3f, 0x46da, 0xa8, 0x28, 0xa3, 0xb4, \
0x57, 0xa4, 0x42, 0x82}
This is an indicator GUID without any data. It represents
the fact that a PEIM has written the address of the EFI_PEI_CPU_IO_PPI
into the EFI_PEI_SERVICES
table.
PPI Interface Structure
typedef
struct \_EFI_PEI_CPU_IO_PPI {
EFI_PEI_CPU_IO_PPI_ACCESS Mem;
EFI_PEI_CPU_IO_PPI_ACCESS Io;
EFI_PEI_CPU_IO_PPI_IO_READ8 IoRead8;
EFI_PEI_CPU_IO_PPI_IO_READ16 IoRead16;
EFI_PEI_CPU_IO_PPI_IO_READ32 IoRead32;
EFI_PEI_CPU_IO_PPI_IO_READ64 IoRead64;
EFI_PEI_CPU_IO_PPI_IO_WRITE8 IoWrite8;
EFI_PEI_CPU_IO_PPI_IO_WRITE16 IoWrite16;
EFI_PEI_CPU_IO_PPI_IO_WRITE32 IoWrite32;
EFI_PEI_CPU_IO_PPI_IO_WRITE64 IoWrite64;
EFI_PEI_CPU_IO_PPI_MEM_READ8 MemRead8;
EFI_PEI_CPU_IO_PPI_MEM_READ16 MemRead16;
EFI_PEI_CPU_IO_PPI_MEM_READ32 MemRead32;
EFI_PEI_CPU_IO_PPI_MEM_READ64 MemRead64;
EFI_PEI_CPU_IO_PPI_MEM_WRITE8 MemWrite8;
EFI_PEI_CPU_IO_PPI_MEM_WRITE16 MemWrite16;
EFI_PEI_CPU_IO_PPI_MEM_WRITE32 MemWrite32;
EFI_PEI_CPU_IO_PPI_MEM_WRITE64 MemWrite64;
} EFI_PEI_CPU_IO_PPI;
Parameters
Mem
Collection of memory-access services. See the
Mem()
function description. TypeEFI_PEI_CPU_IO_PPI_ACCESS
is defined in “Related Definitions” below.
Io
Collection of I/O-access services. See the
Io()
function description. TypeEFI_PEI_CPU_IO_PPI_ACCESS
is defined in “Related Definitions” below.
IoRead8
8-bit read service. See the
IoRead8()
function description.
IoRead16
16-bit read service. See the
IoRead16()
function description.
IoRead32
32-bit read service. See the
IoRead32()
function description.
IoRead64
64-bit read service. See the
IoRead64()
function description.
IoWrite8
8-bit write service. See the
IoWrite8()
function description.
IoWrite16
16-bit write service. See the
IoWrite16()
function description.
IoWrite32
32-bit write service. See the
IoWrite32()
function description.
IoWrite64
64-bit write service. See the
IoWrite64()
function description.
MemRead8
8-bit read service. See the
MemRead8()
function description.
MemRead16
16-bit read service. See the
MemRead16()
function description.
MemRead32
32-bit read service. See the
MemRead32()
function description.
MemRead64
64-bit read service. See the
MemRead64()
function description.
MemWrite8
8-bit write service. See the
MemWrite8()
function description.
MemWrite16
16-bit write service. See the
MemWrite16()
function description.
MemWrite32
32-bit write service. See the
MemWrite32()
function description.
MemWrite64
64-bit write service. See the
MemWrite64()
function description.
Description
This PPI provides a set of memory- and I/O-based services. The perspective of the services is that of the processor, not the bus or system.
//******************************************************\*
// EFI_PEI_CPU_IO_PPI_ACCESS
//******************************************************\*
typedef
struct {
EFI_PEI_CPU_IO_PPI_IO_MEM Read;
EFI_PEI_CPU_IO_PPI_IO_MEM Write;
} EFI_PEI_CPU_IO_PPI_ACCESS;
Read
This service provides the various modalities of memory and I/O read.
Write
This service provides the various modalities of memory and I/O write.
8.3.8.2. EFI_PEI_CPU_IO_PPI.Mem()¶
Summary
Memory-based access services.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_CPU_IO_PPI_IO_MEM) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN EFI_PEI_CPU_IO_PPI_WIDTH Width,
IN UINT64 Address,
IN UINTN Count,
IN OUT VOID *Buffer
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Width
The width of the access. Enumerated in bytes. Type
EFI_PEI_CPU_IO_PPI_WIDTH
is defined in “Related Definitions” below.
Address
The physical address of the access.
Count
The number of accesses to perform.
Buffer
A pointer to the buffer of data.
Description
The Mem()
function provides a list of memory-based
accesses.
//******************************************************\*
// EFI_PEI_CPU_IO_PPI_WIDTH
//******************************************************\*
typedef enum {
EfiPeiCpuIoWidthUint8,
EfiPeiCpuIoWidthUint16,
EfiPeiCpuIoWidthUint32,
EfiPeiCpuIoWidthUint64,
EfiPeiCpuIoWidthFifoUint8,
EfiPeiCpuIoWidthFifoUint16,
EfiPeiCpuIoWidthFifoUint32,
EfiPeiCpuIoWidthFifoUint64,
EfiPeiCpuIoWidthFillUint8,
EfiPeiCpuIoWidthFillUint16,
EfiPeiCpuIoWidthFillUint32,
EfiPeiCpuIoWidthFillUint64,
EfiPeiCpuIoWidthMaximum
} EFI_PEI_CPU_IO_PPI_WIDTH;
Status Codes Returned
EFI_SUCCESS |
The function completed successfully. |
EFI_NOT_YET_AVAILABLE |
The service has not been installed. |
8.3.8.3. EFI_PEI_CPU_IO_PPI.Io()¶
Summary
I/O-based access services.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_CPU_IO_PPI_IO_MEM) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN EFI_PEI_CPU_IO_PPI_WIDTH Width,
IN UINT64 Address,
IN UINTN Count,
IN OUT VOID *Buffer
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Width
The width of the access. Enumerated in bytes. Type
EFI_PEI_CPU_IO_PPI_WIDTH
is defined inMem()
.
Address
The physical address of the access.
Count
The number of accesses to perform.
Buffer
A pointer to the buffer of data.
Description
The Io()
function provides a list of I/O-based accesses.
Input or output data can be found in the last argument.
Status Codes Returned
EFI_SUCCESS |
The function completed successfully. |
EFI_NOT_YET_AVAILABLE |
The service has not been installed. |
8.3.8.4. EFI_PEI_CPU_IO_PPI.IoRead8()¶
Summary
8-bit I/O read operations.
Prototype
typedef
UINT8
(EFIAPI *EFI_PEI_CPU_IO_PPI_IO_READ8) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Description
The IoRead8()
function returns an 8-bit value from the I/O
space.
8.3.8.5. EFI_PEI_CPU_IO_PPI.IoRead16()¶
Summary
16-bit I/O read operations.
Prototype
typedef
UINT16
(EFIAPI *EFI_PEI_CPU_IO_PPI_IO_READ16) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Description
The IoRead16()
function returns a 16-bit value from the
I/O space.
8.3.8.6. EFI_PEI_CPU_IO_PPI.IoRead32()¶
Summary
32-bit I/O read operations.
Prototype
typedef
UINT32
(EFIAPI *EFI_PEI_CPU_IO_PPI_IO_READ32) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Description
The IoRead32()
function returns a 32-bit value from the
I/O space.
8.3.8.7. EFI_PEI_CPU_IO_PPI.IoRead64()¶
Summary
64-bit I/O read operations.
Prototype
typedef
UINT64
(EFIAPI *EFI_PEI_CPU_IO_PPI_IO_READ64) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST_EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Description
The IoRead64()
function returns a 64-bit value from the
I/O space.
8.3.8.8. EFI_PEI_CPU_IO_PPI.IoWrite8()¶
Summary
8-bit I/O write operations.
Prototype
typedef
VOID
(EFIAPI *EFI_PEI_CPU_IO_PPI_IO_WRITE8) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST_EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address,
IN UINT8 Data
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Data
The data to write.
Description
The IoWrite8()
function writes an 8-bit value to the I/O
space.
8.3.8.9. EFI_PEI_CPU_IO_PPI.IoWrite16()¶
Summary
16-bit I/O write operation.
Prototype
typedef
VOID
(EFIAPI *EFI_PEI_CPU_IO_PPI_IO_WRITE16) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address,
IN UINT16 Data
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Data
The data to write.
Description
The IoWrite16()
function writes a 16-bit value to the I/O
space.
8.3.8.10. EFI_PEI_CPU_IO_PPI.IoWrite32()¶
Summary
32-bit I/O write operation.
Prototype
typedef
VOID
(EFIAPI *EFI_PEI_CPU_IO_PPI_IO_WRITE32) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address,
IN UINT32 Data
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Data
The data to write.
Description
The IoWrite32()
function writes a 32-bit value to the I/O
space.
8.3.8.11. EFI_PEI_CPU_IO_PPI.IoWrite64()¶
Summary
64-bit I/O write operation.
Prototype
typedef
VOID
(EFIAPI *EFI_PEI_CPU_IO_PPI_IO_WRITE64) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address,
IN UINT64 Data
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Data
The data to write.
Description
The IoWrite64()
function writes a 64-bit value to the I/O
space.
8.3.8.12. EFI_PEI_CPU_IO_PPI.MemRead8()¶
Summary
8-bit memory read operations.
Prototype
typedef
UINT8
(EFIAPI *EFI_PEI_CPU_IO_PPI_MEM_READ8) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Description
The MemRead8()
function returns an 8-bit value from the
memory space.
8.3.8.13. EFI_PEI_CPU_IO_PPI.MemRead16()¶
Summary
16-bit memory read operations.
Prototype
typedef
UINT16
(EFIAPI *EFI_PEI_CPU_IO_PPI_MEM_READ16) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Description
The MemRead16()
function returns a 16-bit value from the
memory space.
8.3.8.14. EFI_PEI_CPU_IO_PPI.MemRead32()¶
Summary
32-bit memory read operations.
Prototype
typedef
UINT32
(EFIAPI *EFI_PEI_CPU_IO_PPI_MEM_READ32) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Description
The MemRead32()
function returns a 32-bit value from the
memory space.
8.3.8.15. EFI_PEI_CPU_IO_PPI.MemRead64()¶
Summary
64-bit memory read operations.
Prototype
typedef
UINT64
(EFIAPI *EFI_PEI_CPU_IO_PPI_MEM_READ64) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Description
The MemRead64()
function returns a 64-bit value from the
memory space.
8.3.8.16. EFI_PEI_CPU_IO_PPI.MemWrite8()¶
Summary
8-bit memory write operations.
Prototype
typedef
VOID
(EFIAPI *EFI_PEI_CPU_IO_PPI_MEM_WRITE8) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address,
IN UINT8 Data
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Data
The data to write.
Description
The
MemWrite8()
function writes an 8-bit value to the memory space.
8.3.8.17. EFI_PEI_CPU_IO_PPI.MemWrite16()¶
Summary
16-bit memory write operation.
Prototype
typedef
VOID
(EFIAPI *EFI_PEI_CPU_IO_PPI_MEM_WRITE16) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address,
IN UINT16 Data
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Data
The data to write.
Description
The MemWrite16()
function writes a 16-bit value to the
memory space.
8.3.8.18. EFI_PEI_CPU_IO_PPI.MemWrite32()¶
Summary
32-bit memory write operation.
Prototype
typedef
VOID
(EFIAPI *EFI_PEI_CPU_IO_PPI_MEM_WRITE32) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address,
IN UINT32 Data
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Data
The data to write.
Description
The MemWrite32()
function writes a 32-bit value to the
memory space.
8.3.8.19. EFI_PEI_CPU_IO_PPI.MemWrite64()¶
Summary
64-bit memory write operation.
Prototype
typedef
VOID
(EFIAPI *EFI_PEI_CPU_IO_PPI_IO_WRITE64) (
IN CONST EFI_PEI_SERVICES **PeiServices,
IN CONST EFI_PEI_CPU_IO_PPI *This,
IN UINT64 Address,
IN UINT64 Data
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to local data for the interface.
Address
The physical address of the access.
Data
The data to write.
Description
The MemWrite64()
function writes a 64-bit value to the
memory space.
//
// Vector Handoff Info Attributes
//
#define EFI_VECTOR_HANDOFF_DO_NOT_HOOK 0x00000000
#define EFI_VECTOR_HANDOFF_HOOK_BEFORE 0x00000001
#define EFI_VECTOR_HANDOFF_HOOK_AFTER 0x00000002
#define EFI_VECTOR_HANDOFF_LAST_ENTRY 0x80000000
8.3.9. EFI Pei Capsule PPI¶
8.3.9.1. EFI_PEI_CAPSULE_PPI (Optional)¶
Summary
This PPI is installed by some platform or chipset-specific PEIM that abstracts handling of UEFI Capsule processing.
GUID
#define EFI_PEI_CAPSULE_PPI_GUID \
{0x3acf33ee, 0xd892, 0x40f4, \
{0xa2, 0xfc, 0x38, 0x54, 0xd2, 0xe1, 0x32, 0x3d } }
PPI Interface Structure
typedef
struct \_EFI_PEI_CAPSULE_PPI {
EFI_PEI_CAPSULE_COALESCE Coalesce;
EFI_PEI_CAPSULE_CHECK_CAPSULE_UDPATE CheckCapsuleUpdate;
EFI_PEI_CAPSULE_CREATE_STATE CreateState;
} EFI_PEI_CAPSULE_PPI;
Parameters
Coalesce
Upon determining that there is a capsule to operate on, this service will use a series of
EFI_CAPSULE_BLOCK_DESCRIPTOR
entries to determine the current location of the various capsule fragments and coalesce them into a contiguous region of system memory.
CheckCapsuleUpdate
Determine if a capsule needs to be processed. The means by which the presence of a capsule is determined is platform specific. For example, an implementation could be driven by the presence of a Capsule EFI Variable containing a list of
EFI_CAPSULE_BLOCK_DESCRIPTOR
entries. If present, returnEFI_SUCCESS
, otherwise returnEFI_NOT_FOUND
.
CreateState
The Capsule PPI service that gets called after memory is available. The capsule coalesce function, which must be called first, returns a base address and size. Once the memory init PEIM has discovered memory, it should call this function and pass in the base address and size returned by the Coalesce() function. Then this function can create a capsule HOB and return.
Description
This PPI provides several services in PEI to work with the underlying capsule capabilities of the platform. These services include the ability for PEI to coalesce a capsule from a scattered set of memory locations into a contiguous space in memory, detect if a capsule is present for processing, and once memory is available, create a HOB for the capsule.
8.3.9.2. EFI_PEI_CAPSULE_PPI.Coalesce¶
Summary
Coalesce the capsule
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_CAPSULE_COALESCE)(
IN EFI_PEI_SERVICES **PeiServices,
IN OUT VOID *MemoryBase,
IN OUT UINTN *MemSize
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
MemoryBase
Pointer to the base of a block of memory into which the buffers will be coalesced. On output, this variable will hold the base address of a coalesced capsule.
MemorySize
Pointer to local data for the interface.
Description
Upon determining that there is a capsule to operate on, this
service will use a series of EFI_CAPSULE_BLOCK_DESCRIPTOR
entries to determine the current location of the various
capsule fragments and coalesce them into a contiguous region
of system memory.
Status Codes Returned
EFI_SUCCESS |
There was no capsule or the capsule was processed successfully |
EFI_NOT_FOUND |
If boot mode could not be determined or the boot mode is not flash update or the capsule descriptors were not found |
EFI_BUFFER_TOO_SMALL |
The capsule could not be coalesced in the provided memory region |
8.3.9.3. EFI_PEI_CAPSULE_CHECK_CAPSULE_UDPATE.CheckCapsuleUpdate()¶
Summary
Check the Capsule Update.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_CAPSULE_CHECK_CAPSULE_UPDATE)(
IN EFI_PEI_SERVICES **PeiServices
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
Description
Determine if a capsule needs to be processed. The means by
which the presence of a capsule is determined is platform
specific. For example, an implementation could be driven by
the presence of a Capsule EFI Variable containing a list of
EFI_CAPSULE_BLOCK_DESCRIPTOR
entries. If present, return
EFI_SUCCESS
, otherwise return EFI_NOT_FOUND
.
Status Codes Returned
EFI_SUCCESS |
If a capsule is available. |
EFI_NOT_FOUND |
No capsule detected. |
8.3.9.4. EFI_PEI_CAPSULE_CHECK_CAPSULE_UDPATE.CapsuleCreateState()¶
Summary
Create the Capsule state.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_CAPSULE_CREATE_STATE)(
IN EFI_PEI_SERVICES **PeiServices,
IN VOID *CapsuleBase,
IN UINTN CapsuleSize
);
Parameters
PeiServices
Pointer to the PEI Services Table.
CapsuleBase
Address returned by the capsule coalesce function.
CapsuleSize
Value returned by the capsule coalesce function.
Description
The Capsule PPI service that gets called after memory is available. The capsule coalesce function, which must be called first, returns a base address and size. Once the memory init PEIM has discovered memory, it should call this function and pass in the base address and size returned by the Coalesce() function. Then this function can create a capsule HOB and return.
Status Codes Returned
EFI_VOLUME_CORRUPTED |
|
EFI_SUCCESS |
Capsule HOB was created successfully |
8.3.9.5. EFI_PEI_MP_SERVICES_PPI (Optional)¶
Summary
This PPI is installed by some platform or chipset-specific PEIM that abstracts handling multiprocessor support.
GUID
#define EFI_PEI_MP_SERVICES_PPI_GUID \
{ 0xee16160a, 0xe8be, 0x47a6, { 0x82, 0xa, 0xc6, 0x90, 0xd, 0xb0, 0x25, 0xa } }
PPI Interface Structure
typedef
struct _EFI_PEI_MP_SERVICES_PPI {
EFI_PEI_MP_SERVICES_GET_NUMBER_OF_PROCESSORS GetNumberOfProcessors;
EFI_PEI_MP_SERVICES_GET_PROCESSOR_INFO GetProcessorInfo;
EFI_PEI_MP_SERVICES_STARTUP_ALL_APS StartupAllAPs;
EFI_PEI_MP_SERVICES_STARTUP_THIS_AP StartupThisAP;
EFI_PEI_MP_SERVICES_SWITCH_BSP SwitchBSP;
EFI_PEI_MP_SERVICES_ENABLEDISABLEAP EnableDisableAP;
EFI_PEI_MP_SERVICES_WHOAMI WhoAmI;
} EFI_PEI_MP_SERVICES_PPI;
Parameters
GetNumberOfProcessors
Discover the number of CPU’s
GetProcessorInfo
Ascertain information on the CPU’s.
StartupAllAPs
Startup all of the application processors.
StartupThisAP
Startup the specific application processor.
SwitchBSP
Switch the boot strap processor.
WhoAmI
Identify the currently executing processor.
Description
When installed, the MP Services PPI produces a collection of services that are needed for MP management.
Before the PI event END_OF_PEI
is signaled, the module
that produces this protocol is required to place all APs
into an idle state whenever the APs are disabled or the APs
are not executing code as requested through the
StartupAllAPs()
or StartupThisAP()
services. The idle
state of an AP before the PI event END_OF_PEI
is signaled
is implementation dependent.
After the PI event END_OF_PEI
is signaled, all the APs
must be placed in the OS compatible CPU state as defined by
the UEFI Specification
. Implementations of this Ppi may
use the PI event END_OF_PEI
to force APs into the OS
compatible state as defined by the UEFI Specification.
The support for SwitchBSP()
and EnableDisableAP()
may no
longer be supported after the PEI event END_OF_PEI
is
signaled.
8.3.9.6. EFI_PEI_MP_SERVICES_PPI.GetNumberOfProcessors()¶
Summary
Get the number of CPUs
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_MP_SERVICES_GET_NUMBER_OF_PROCESSORS)(
IN CONST EFI_PEI_SERVICES **PeiServices,
IN EFI_PEI_MP_SERVICES_PPI *This,
OUT UINTN *NumberOfProcessors,
OUT UINTN *NumberOfEnabledProcessors
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
Pointer to this instance of the PPI.
NumberOfProcessors
Pointer to the total number of logical processors in the system, including the BSP and disabled APs.
NumberOfEnabledProcessors
Number of processors in the system that are enabled.
Description
This service retrieves the number of logical processor in the platform and the number of those logical processors that are enabled on this boot. This service may only be called from the BSP.
This function is used to retrieve the following information:
The number of logical processors that are present in the system.
The number of enabled logical processors in the system at the instant this call is made.
Because MP Service PPI provides services to enable and disable processors dynamically, the number of enabled logical processors may vary during the course of a boot session.
If this service is called from an AP, then
EFI_DEVICE_ERROR
is returned.
If NumberOfProcessors
or NumberOfEnabledProcessors
is
NULL, then EFI_INVALID_PARAMETER
is returned. Otherwise,
the total number of processors is returned in
NumberOfProcessors
, the number of currently enabled
processor is returned in NumberOfEnabledProcessors
, and
EFI_SUCCESS
is returned.
Status Codes Returned
EFI_SUCCESS |
The number of logical processors and enabled logical processors was retrieved |
EFI_DEVICE_ERROR |
The calling processor is an AP |
EFI_INVALID_PARAMETER |
|
EFI_INVALID_PARAMETER |
|
8.3.9.7. EFI_PEI_MP_SERVICES_PPI.GetProcessorInfo()¶
Summary
Get information on a specific CPU.
Prototype
typedef
EFI_STATUS
(EFIAPI EFI_PEI_MP_SERVICES_GET_PROCESSOR_INFO)(
IN CONST EFI_PEI_SERVICES **PeiServices,
IN EFI_PEI_MP_SERVICES_PPI *This,
IN UINTN ProcessorNumber,
OUT EFI_PROCESSOR_INFORMATION *ProcessorInfoBuffer
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
A pointer to the
EFI_PEI_MP_SERVICES_PPI
instance.ProcessorNumber
The handle number of the processor.
ProcessorInfoBuffer
A pointer to the buffer where the processor information is stored.
Description
Gets detailed MP-related information on the requested processor at the instant this call is made. This service may only be called from the BSP.
This service retrieves detailed MP-related information about any processor on the platform. Note the following:
The processor information may change during the course of a boot session.
The information presented here is entirely MP related.
Information regarding the number of caches and their sizes, frequency of operation, slot numbers is all considered platform-related information and is not provided by this service.
Status Codes Returned
EFI_SUCCESS |
Processor information was returned |
EFI_DEVICE_ERROR |
The calling processor is an AP |
EFI_INVALID_PARAMETER |
|
EFI_NOT_FOUND |
The processor with the handle specified by |
8.3.9.8. EFI_PEI_MP_SERVICES_PPI.StartupAllAPs()¶
Summary
Activate all of the application proessors.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_MP_SERVICES_STARTUP_ALL_APS)(
IN CONST EFI_PEI_SERVICES **PeiServices,
IN EFI_PEI_MP_SERVICES_PPI *This,
IN EFI_AP_PROCEDURE Procedure,
IN BOOLEAN SingleThread,
IN UINTN TimeoutInMicroSeconds,
IN VOID *ProcedureArgument OPTIONAL
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
A pointer to the
EFI_PEI_MP_SERVICES_PPI
instance.Procedure
A pointer to the function to be run on enabled APs of the system. See type
EFI_AP_PROCEDURE
.SingleThread
If
TRUE
, then all the enabled APs execute the function specified byProcedure
one by one, in ascending order of processor handle number. IfFALSE
, then all the enabled APs execute the function specified byProcedure
simultaneously.TimeoutInMicroseconds
Indicates the time limit in microseconds for APs to return from Procedure, for blocking mode only. Zero means infinity. If the timeout expires before all APs return from
Procedure
, thenProcedure
on the failed APs is terminated. All enabled APs are available for next function assigned byEFI_PEI_MP_SERVICES_PPI.StartupAllAPs()
orEFI_PEI_MP_SERVICES_PPI.StartupThisAP()
.If the timeout expires in blocking mode, BSP returns
EFI_TIMEOUT
.ProcedureArgument
The parameter passed into
Procedure
for all APs.
Description
This service executes a caller provided function on all enabled APs. APs can run either simultaneously or one at a time in sequence. This service supports both blocking requests only. This service may only be called from the BSP.
This function is used to dispatch all the enabled APs to the
function specified by Procedure
. If any enabled AP is
busy, then EFI_NOT_READY
is returned immediately and
Procedure
is not started on any AP.
If SingleThread
is TRUE
, all the enabled APs execute
the function specified by Procedure
one by one, in
ascending order of processor handle number. Otherwise, all
the enabled APs execute the function specified by
Procedure
simultaneously.
If the timeout specified by TimeoutInMicroseconds
expires
before all APs return from Procedure
, then Procedure
on
the failed APs is terminated. All enabled APs are always
available for further calls to
EFI_PEI_MP_SERVICES_PPI.StartupAllAPs()
and
EFI_PEI_MP_SERVICES_PPI.StartupThisAP()
. If FailedCpuList
is not NULL, its content points to the list of processor
handle numbers in which Procedure
was terminated.
Note: It is the responsibility of the consumer of the
EFI_PEI_MP_SERVICES_PPI.StartupAllAPs()
to make sure that
the nature of the code that is executed on the BSP and
the dispatched APs is well controlled. The MP Services
Ppi does not guarantee that the Procedure
function is
MP-safe. Hence, the tasks that can be run in parallel are
limited to certain independent tasks and well-controlled
exclusive code. PEI services and Ppis may not be called
by APs unless otherwise specified.
In blocking execution mode, BSP waits until all APs finish
or TimeoutInMicroSeconds
expires.
Status Codes Returned
EFI_SUCCESS |
In blocking mode all APs have finished before the timeout expired |
EFI_DEVICE_ERROR |
Caller processor is AP |
EFI_NOT_STARTED |
No enabled APs exist in the system |
EFI_NOT_READY |
Any enabled APs are busy |
EFI_TIMEOUT |
In blocking mode the timeout expired before all enabled APs have finished |
EFI_INVALID_PARAMETER |
|
8.3.9.9. EFI_PEI_MP_SERVICES_PPI.StartupThisAP ()¶
Summary
Activate a specific application processor
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_MP_SERVICES_STARTUP_THIS_AP)(
IN CONST EFI_PEI_SERVICES **PeiServices,
IN EFI_PEI_MP_SERVICES_PPI *This,
IN EFI_AP_PROCEDURE Procedure,
IN UINTN ProcessorNumber,
IN UINTN TimeoutInMicroseconds,
IN VOID *ProcedureArgument OPTIONAL
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
A pointer to the
EFI_PEI_MP_SERVICES_PPI
instance.Procedure
A pointer to the function to be run on enabled APs of the system. See type
EFI_AP_PROCEDURE
.ProcessorNumber
The handle number of the AP. The range is from 0 to the total number of logical processors minus 1. The total number of logical processors can be retrieved by
EFI_PEI_MP_SERVICES_PPI.GetNumberOfProcessors()
.TimeoutInMicrosecsond
Indicates the time limit in microseconds for APs to return from
Procedure
, for blocking mode only. Zero means infinity. If the timeout expires before all APs return fromProcedure
, thenProcedure
on the failed APs is terminated. All enabled APs are available for next function assigned byEFI_PEI_MP_SERVICES_PPI.StartupAllAPs()
orEFI_PEI_MP_SERVICES_PPI.StartupThisAP()
.If the timeout expires in blocking mode, BSP returns
EFI_TIMEOUT
.ProcedureArgument
The parameter passed into Procedure for all APs.
Description
This service lets the caller get one enabled AP to execute a caller-provided function. The caller can request the BSP to wait for the completion of the AP. This service may only be called from the BSP.
This function is used to dispatch one enabled AP to the
function specified by Procedure
passing in the argument
specified by ProcedureArgument
.
The execution is in blocking mode. The BSP waits until the
AP finishes or TimeoutInMicroSecondss
expires.
If the timeout specified by TimeoutInMicroseconds
expires
before the AP returns from Procedure
, then execution of
Procedure
by the AP is terminated. The AP is available for
subsequent calls to EFI_PEI_MP_SERVICES_PPI.StartupAllAPs()
and EFI_PEI_MP_SERVICES_PPI.StartupThisAP()
.
Status Codes Returned
EFI_SUCCESS |
In blocking mode specified AP finished before the timeout expires |
EFI_DEVICE_ERROR |
The calling processor is an AP |
EFI_TIMEOUT |
In blocking mode the timeout expired before the specified AP has finished |
EFI_NOT_FOUND |
The processor with the handle specified by |
EFI_INVALID_PARAMETER |
|
EFI_INVALID_PARAMETER |
|
8.3.9.10. EFI_PEI_MP_SERVICES_PPI.SwitchBSP ()¶
Summary
Switch the boot strap processor
Prototype
typedef
(EFIAPI *EFI_PEI_MP_SERVICES_SWITCH_BSP)(
IN CONST EFI_PEI_SERVICES **PeiServices,
IN EFI_PEI_MP_SERVICES_PPI *This,
IN UINTN *ProcessorNumber,
IN BOOLEAN *EnableOldBSP
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
A pointer to the
EFI_PEI_MP_SERVICES_PPI
instance.ProcessorNumber
The handle number of AP that is to become the new BSP. The range is from 0 to the total number of logical processors minus 1. The total number of logical processors can be retrieved by
EFI_PEI_MP_SERVICES_PPI.GetNumberOfProcessors()
.EnableOldBSP
If
TRUE
, then the old BSP will be listed as an enabled AP. Otherwise, it will be disabled.
Description
This service switches the requested AP to be the BSP from that point onward.
This service changes the BSP for all purposes.This call can only be performed by the current BSP.
This service switches the requested AP to be the BSP from that point onward. This service changes the BSP for all purposes. The new BSP can take over the execution of the old BSP and continue seamlessly from where the old one left off.
If the BSP cannot be switched prior to the return from this
service, then EFI_UNSUPPORTED
must be returned.
Status Codes Returned
EFI_SUCCESS |
BSP successfully switched |
EFI_UNSUPPORTED |
Switching the BSP cannot be completed prior to this service returning |
EFI_UNSUPPORTED |
Switching the BSP is not supported |
EFI_DEVICE_ERROR |
The calling processor is an AP |
EFI_NOT_FOUND |
The processor with the handle specified by |
EFI_INVALID_PARAMETER |
|
EFI_NOT_READY |
The specified AP is busy |
Summary
Switch the boot strap processor
Prototype
typedef
(EFIAPI *EFI_PEI_MP_SERVICES_ENABLEDISABLEAP)(
IN CONST EFI_PEI_SERVICES **PeiServices,
IN EFI_PEI_MP_SERVICES_PPI *This,
IN UINTN ProcessorNumber,
IN BOOLEAN EnableAP,
IN UINT32 *HealthFlag OPTIONAL
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
A pointer to the
EFI_PEI_MP_SERVICES_PPI
instance.ProcessorNumber
The handle number of AP that is to become the new BSP. The range is from 0 to the total number of logical processors minus 1. The total number of logical processors can be retrieved by
EFI_PEI_MP_SERVICES_PPI.GetNumberOfProcessors()
.EnableAP
Specifies the new state for the processor for enabled, FALSE for disabled.
HealthFlag
If not NULL, a pointer to a value that specifies the new health status of the AP. This flag corresponds to
StatusFlag
defined inEFI_PEI_MP_SERVICES_PPI.GetProcessorInfo()
. Only thePROCESSOR_HEALTH_STATUS_BIT
is used. All other bits are ignored. If it is NULL, this parameter is ignored.
Description
This service lets the caller enable or disable an AP from this point onward.
This service may only be called from the BSP.
This service allows the caller enable or disable an AP from this point onward. The caller can optionally specify the health status of the AP by Health. If an AP is being disabled, then the state of the disabled AP is implementation dependent. If an AP is enabled, then the implementation must guarantee that a complete initialization sequence is performed on the AP, so the AP is in a state that is compatible with an MP operating system.
If the enable or disable AP operation cannot be completed
prior to the return from this service, then
EFI_UNSUPPORTED
must be returned.
Status Codes Returned
EFI_SUCCESS |
The specified AP was enabled or disabled successfully |
EFI_UNSUPPORTED |
Enabling or disabling an AP cannot be completed prior to this service returning |
EFI_UNSUPPORTED |
Enabling or disabling an AP is not supported |
EFI_DEVICE_ERROR |
The calling processor is an AP |
EFI_NOT_FOUND |
Processor with the handle specified by |
EFI_INVALID_PARAMETER |
|
8.3.9.11. EFI_PEI_MP_SERVICES_PPI.WhoAmI ()¶
Summary
Identify the currently executing processor.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_MP_SERVICES_WHOAMI)(
IN CONST EFI_PEI_SERVICES **PeiServices,
IN EFI_PEI_MP_SERVICES_PPI *This,
OUT UINTN *ProcessorNumber
);
Parameters
PeiServices
An indirect pointer to the PEI Services Table published by the PEI Foundation.
This
A pointer to the
EFI_PEI_MP_SERVICES_PPI
instance.ProcessorNumber
The handle number of AP that is to become the new BSP. The range is from 0 to the total number of logical processors minus 1. The total number of logical processors can be retrieved by
EFI_PEI_MP_SERVICES_PPI.GetNumberOfProcessors()
.
Descriptions
This services returns the handle number for the calling processor. This service may be called from the BSP and APs.
This service returns the processor handle number for the calling processor.
The returned value is in the range from 0 to the total
number of logical processors minus 1. The total number of
logical processors can be retrieved with
EFI_PEI_MP_SERVICES_PPI.GetNumberOfProcessors()
. This service
may be called from the BSP and APs. If ProcessorNumber
is
NULL, then EFI_INVALID_PARAMETER
is returned. Otherwise,
the current processors handle number is returned in
ProcessorNumber
, and EFI_SUCCESS
is returned.
Status Codes Returned
EFI_SUCCESS |
The current processor handle number was returned in |
EFI_INVALID_PARAMETER |
|
8.3.9.12. EDKII_PEI_MP_SERVICES2_PPI (Optional)¶
Summary
This PPI is installed by some platform or chipset-specific PEIM that abstracts handling multiprocessor support.
GUID
#define EDKII_PEI_MP_SERVICES2_PPI_GUID \
{ 0x5cb9cb3d, 0x31a4, 0x480c, { 0x94, 0x98, 0x29, 0xd2, 0xb9, 0xba, 0xcf, 0xba } }
PPI Interface Structure
typedef
struct _EDKII_PEI_MP_SERVICES2_PPI {
EDKII_PEI_MP_SERVICES_GET_NUMBER_OF_PROCESSORS GetNumberOfProcessors;
EDKII_PEI_MP_SERVICES_GET_PROCESSOR_INFO GetProcessorInfo;
EDKII_PEI_MP_SERVICES_STARTUP_ALL_APS StartupAllAPs;
EDKII_PEI_MP_SERVICES_STARTUP_THIS_AP StartupThisAP;
EDKII_PEI_MP_SERVICES_SWITCH_BSP SwitchBSP;
EDKII_PEI_MP_SERVICES_ENABLEDISABLEAP EnableDisableAP;
EDKII_PEI_MP_SERVICES_WHOAMI WhoAmI;
EDKII_PEI_MP_SERVICES_STARTUP_ALL_CPUS StartupAllCPUs;
} EDKII_PEI_MP_SERVICES2_PPI;
Parameters
GetNumberOfProcessors
Discover the number of CPU’s
GetProcessorInfo
Ascertain information on the CPU’s.
StartupAllAPs
Startup all of the application processors.
StartupThisAP
Startup the specific application processor.
SwitchBSP
Switch the boot strap processor.
WhoAmI
Identify the currently executing processor.
StartupAllCPUs
Description
When installed, the MP Services PPI produces a collection of services that are needed for MP management.
Before the PI event END_OF_PEI
is signaled, the module
that produces this protocol is required to place all APs
into an idle state whenever the APs are disabled or the APs
are not executing code as requested through the
StartupAllAPs()
or StartupThisAP()
services. The idle
state of an AP before the PI event END_OF_PEI
is signaled
is implementation dependent.
After the PI event END_OF_PEI
is signaled, all the APs
must be placed in the OS compatible CPU state as defined by
the UEFI Specification
. Implementations of this Ppi may
use the PI event END_OF_PEI
to force APs into the OS
compatible state as defined by the UEFI Specification.
The support for SwitchBSP()
and EnableDisableAP()
may no
longer be supported after the PEI event END_OF_PEI
is
signaled.
8.3.9.13. EDKII_PEI_MP_SERVICES2_PPI.GetNumberOfProcessors()¶
Summary
Get the number of CPUs
Prototype
typedef
EFI_STATUS
(EFIAPI *EDKII_PEI_MP_SERVICES_GET_NUMBER_OF_PROCESSORS)(
IN EDKII_PEI_MP_SERVICES2_PPI *This,
OUT UINTN *NumberOfProcessors,
OUT UINTN *NumberOfEnabledProcessors
);
Parameters
This
Pointer to this instance of the PPI.
NumberOfProcessors
Pointer to the total number of logical processors in the system, including the BSP and disabled APs.
NumberOfEnabledProcessors
Number of processors in the system that are enabled.
Description
This service retrieves the number of logical processor in the platform and the number of those logical processors that are enabled on this boot. This service may only be called from the BSP.
This function is used to retrieve the following information:
The number of logical processors that are present in the system.
The number of enabled logical processors in the system at the instant this call is made.
Because MP Service PPI provides services to enable and disable processors dynamically, the number of enabled logical processors may vary during the course of a boot session.
If this service is called from an AP, then
EFI_DEVICE_ERROR
is returned.
If NumberOfProcessors
or NumberOfEnabledProcessors
is
NULL, then EFI_INVALID_PARAMETER
is returned. Otherwise,
the total number of processors is returned in
NumberOfProcessors
, the number of currently enabled
processor is returned in NumberOfEnabledProcessors
, and
EFI_SUCCESS
is returned.
Status Codes Returned
EFI_SUCCESS |
The number of logical processors and enabled logical processors was retrieved |
EFI_DEVICE_ERROR |
The calling processor is an AP |
EFI_INVALID_PARAMETER |
|
EFI_INVALID_PARAMETER |
|
8.3.9.14. EDKII_PEI_MP_SERVICES2_PPI.GetProcessorInfo()¶
Summary
Get information on a specific CPU.
Prototype
typedef
EFI_STATUS
(EFIAPI *EDKII_PEI_MP_SERVICES_GET_PROCESSOR_INFO)(
IN EDKII_PEI_MP_SERVICES2_PPI *This,
IN UINTN ProcessorNumber,
OUT EFI_PROCESSOR_INFORMATION *ProcessorInfoBuffer
);
Parameters
This
A pointer to the
EDKII_PEI_MP_SERVICES2_PPI
instance.ProcessorNumber
The handle number of the processor.
ProcessorInfoBuffer
A pointer to the buffer where the processor information is stored.
Description
Gets detailed MP-related information on the requested processor at the instant this call is made. This service may only be called from the BSP.
This service retrieves detailed MP-related information about any processor on the platform. Note the following:
The processor information may change during the course of a boot session.
The information presented here is entirely MP related.
Information regarding the number of caches and their sizes, frequency of operation, slot numbers is all considered platform-related information and is not provided by this service.
Status Codes Returned
EFI_SUCCESS |
Processor information was returned |
EFI_DEVICE_ERROR |
The calling processor is an AP |
EFI_INVALID_PARAMETER |
|
EFI_NOT_FOUND |
The processor with the handle specified by |
8.3.9.15. EDKII_PEI_MP_SERVICES2_PPI.StartupAllAPs()¶
Summary
Activate all of the application proessors.
Prototype
typedef
EFI_STATUS
(EFIAPI *EDKII_PEI_MP_SERVICES_STARTUP_ALL_APS)(
IN EDKII_PEI_MP_SERVICES2_PPI *This,
IN EFI_AP_PROCEDURE Procedure,
IN BOOLEAN SingleThread,
IN UINTN TimeoutInMicroSeconds,
IN VOID *ProcedureArgument OPTIONAL
);
Parameters
This
A pointer to the
EDKII_PEI_MP_SERVICES2_PPI
instance.Procedure
A pointer to the function to be run on enabled APs of the system. See type
EFI_AP_PROCEDURE
.SingleThread
If
TRUE
, then all the enabled APs execute the function specified byProcedure
one by one, in ascending order of processor handle number. IfFALSE
, then all the enabled APs execute the function specified byProcedure
simultaneously.TimeoutInMicroseconds
Indicates the time limit in microseconds for APs to return from Procedure, for blocking mode only. Zero means infinity. If the timeout expires before all APs return from
Procedure
, thenProcedure
on the failed APs is terminated. All enabled APs are available for next function assigned byEDKII_PEI_MP_SERVICES2_PPI.StartupAllAPs()
orEDKII_PEI_MP_SERVICES2_PPI.StartupThisAP()
.If the timeout expires in blocking mode, BSP returns
EFI_TIMEOUT
.ProcedureArgument
The parameter passed into
Procedure
for all APs.
Description
This service executes a caller provided function on all enabled APs. APs can run either simultaneously or one at a time in sequence. This service supports both blocking requests only. This service may only be called from the BSP.
This function is used to dispatch all the enabled APs to the
function specified by Procedure
. If any enabled AP is
busy, then EFI_NOT_READY
is returned immediately and
Procedure
is not started on any AP.
If SingleThread
is TRUE
, all the enabled APs execute
the function specified by Procedure
one by one, in
ascending order of processor handle number. Otherwise, all
the enabled APs execute the function specified by
Procedure
simultaneously.
If the timeout specified by TimeoutInMicroseconds
expires
before all APs return from Procedure
, then Procedure
on
the failed APs is terminated. All enabled APs are always
available for further calls to
EDKII_PEI_MP_SERVICES2_PPI.StartupAllAPs()
and
EDKII_PEI_MP_SERVICES2_PPI.StartupThisAP()
. If FailedCpuList
is not NULL, its content points to the list of processor
handle numbers in which Procedure
was terminated.
Note: It is the responsibility of the consumer of the
EDKII_PEI_MP_SERVICES2_PPI.StartupAllAPs()
to make sure that
the nature of the code that is executed on the BSP and
the dispatched APs is well controlled. The MP Services
Ppi does not guarantee that the Procedure
function is
MP-safe. Hence, the tasks that can be run in parallel are
limited to certain independent tasks and well-controlled
exclusive code. PEI services and Ppis may not be called
by APs unless otherwise specified.
In blocking execution mode, BSP waits until all APs finish
or TimeoutInMicroSeconds
expires.
Status Codes Returned
EFI_SUCCESS |
In blocking mode all APs have finished before the timeout expired |
EFI_DEVICE_ERROR |
Caller processor is AP |
EFI_NOT_STARTED |
No enabled APs exist in the system |
EFI_NOT_READY |
Any enabled APs are busy |
EFI_TIMEOUT |
In blocking mode the timeout expired before all enabled APs have finished |
EFI_INVALID_PARAMETER |
|
8.3.9.16. EDKII_PEI_MP_SERVICES2_PPI.StartupThisAP ()¶
Summary
Activate a specific application processor
Prototype
typedef
EFI_STATUS
(EFIAPI *EDKII_PEI_MP_SERVICES_STARTUP_THIS_AP)(
IN EDKII_PEI_MP_SERVICES2_PPI *This,
IN EFI_AP_PROCEDURE Procedure,
IN UINTN ProcessorNumber,
IN UINTN TimeoutInMicroseconds,
IN VOID *ProcedureArgument OPTIONAL
);
Parameters
This
A pointer to the
EDKII_PEI_MP_SERVICES2_PPI
instance.Procedure
A pointer to the function to be run on enabled APs of the system. See type
EFI_AP_PROCEDURE
.ProcessorNumber
The handle number of the AP. The range is from 0 to the total number of logical processors minus 1. The total number of logical processors can be retrieved by
EDKII_PEI_MP_SERVICES2_PPI.GetNumberOfProcessors()
.TimeoutInMicrosecsond
Indicates the time limit in microseconds for APs to return from
Procedure
, for blocking mode only. Zero means infinity. If the timeout expires before all APs return fromProcedure
, thenProcedure
on the failed APs is terminated. All enabled APs are available for next function assigned byEDKII_PEI_MP_SERVICES2_PPI.StartupAllAPs()
orEDKII_PEI_MP_SERVICES2_PPI.StartupThisAP()
.If the timeout expires in blocking mode, BSP returns
EFI_TIMEOUT
.ProcedureArgument
The parameter passed into Procedure for all APs.
Description
This service lets the caller get one enabled AP to execute a caller-provided function. The caller can request the BSP to wait for the completion of the AP. This service may only be called from the BSP.
This function is used to dispatch one enabled AP to the
function specified by Procedure
passing in the argument
specified by ProcedureArgument
.
The execution is in blocking mode. The BSP waits until the
AP finishes or TimeoutInMicroSecondss
expires.
If the timeout specified by TimeoutInMicroseconds
expires
before the AP returns from Procedure
, then execution of
Procedure
by the AP is terminated. The AP is available for
subsequent calls to EDKII_PEI_MP_SERVICES2_PPI.StartupAllAPs()
and EDKII_PEI_MP_SERVICES2_PPI.StartupThisAP()
.
Status Codes Returned
EFI_SUCCESS |
In blocking mode specified AP finished before the timeout expires |
EFI_DEVICE_ERROR |
The calling processor is an AP |
EFI_TIMEOUT |
In blocking mode the timeout expired before the specified AP has finished |
EFI_NOT_FOUND |
The processor with the handle specified by |
EFI_INVALID_PARAMETER |
|
EFI_INVALID_PARAMETER |
|
8.3.9.17. EDKII_PEI_MP_SERVICES2_PPI.SwitchBSP ()¶
Summary
Switch the boot strap processor
Prototype
typedef
(EFIAPI *EDKII_PEI_MP_SERVICES_SWITCH_BSP)(
IN EDKII_PEI_MP_SERVICES2_PPI *This,
IN UINTN ProcessorNumber,
IN BOOLEAN EnableOldBSP
);
Parameters
This
A pointer to the
EDKII_PEI_MP_SERVICES2_PPI
instance.ProcessorNumber
The handle number of AP that is to become the new BSP. The range is from 0 to the total number of logical processors minus 1. The total number of logical processors can be retrieved by
EDKII_PEI_MP_SERVICES2_PPI.GetNumberOfProcessors()
.EnableOldBSP
If
TRUE
, then the old BSP will be listed as an enabled AP. Otherwise, it will be disabled.
Description
This service switches the requested AP to be the BSP from that point onward.
This service changes the BSP for all purposes.This call can only be performed by the current BSP.
This service switches the requested AP to be the BSP from that point onward. This service changes the BSP for all purposes. The new BSP can take over the execution of the old BSP and continue seamlessly from where the old one left off.
If the BSP cannot be switched prior to the return from this
service, then EFI_UNSUPPORTED
must be returned.
Status Codes Returned
EFI_SUCCESS |
BSP successfully switched |
EFI_UNSUPPORTED |
Switching the BSP cannot be completed prior to this service returning |
EFI_UNSUPPORTED |
Switching the BSP is not supported |
EFI_DEVICE_ERROR |
The calling processor is an AP |
EFI_NOT_FOUND |
The processor with the handle specified by |
EFI_INVALID_PARAMETER |
|
EFI_NOT_READY |
The specified AP is busy |
Summary
Switch the boot strap processor
Prototype
typedef
(EFIAPI *EDKII_PEI_MP_SERVICES_ENABLEDISABLEAP)(
IN EDKII_PEI_MP_SERVICES2_PPI *This,
IN UINTN ProcessorNumber,
IN BOOLEAN EnableAP,
IN UINT32 *HealthFlag OPTIONAL
);
Parameters
This
A pointer to the
EDKII_PEI_MP_SERVICES2_PPI
instance.ProcessorNumber
The handle number of AP that is to become the new BSP. The range is from 0 to the total number of logical processors minus 1. The total number of logical processors can be retrieved by
EDKII_PEI_MP_SERVICES2_PPI.GetNumberOfProcessors()
.EnableAP
Specifies the new state for the processor for enabled, FALSE for disabled.
HealthFlag
If not NULL, a pointer to a value that specifies the new health status of the AP. This flag corresponds to
StatusFlag
defined inEDKII_PEI_MP_SERVICES2_PPI.GetProcessorInfo()
. Only thePROCESSOR_HEALTH_STATUS_BIT
is used. All other bits are ignored. If it is NULL, this parameter is ignored.
Description
This service lets the caller enable or disable an AP from this point onward.
This service may only be called from the BSP.
This service allows the caller enable or disable an AP from this point onward. The caller can optionally specify the health status of the AP by Health. If an AP is being disabled, then the state of the disabled AP is implementation dependent. If an AP is enabled, then the implementation must guarantee that a complete initialization sequence is performed on the AP, so the AP is in a state that is compatible with an MP operating system.
If the enable or disable AP operation cannot be completed
prior to the return from this service, then
EFI_UNSUPPORTED
must be returned.
Status Codes Returned
EFI_SUCCESS |
The specified AP was enabled or disabled successfully |
EFI_UNSUPPORTED |
Enabling or disabling an AP cannot be completed prior to this service returning |
EFI_UNSUPPORTED |
Enabling or disabling an AP is not supported |
EFI_DEVICE_ERROR |
The calling processor is an AP |
EFI_NOT_FOUND |
Processor with the handle specified by |
EFI_INVALID_PARAMETER |
|
8.3.9.18. EDKII_PEI_MP_SERVICES2_PPI.WhoAmI ()¶
Summary
Identify the currently executing processor.
Prototype
typedef
EFI_STATUS
(EFIAPI *EDKII_PEI_MP_SERVICES_WHOAMI)(
IN EDKII_PEI_MP_SERVICES2_PPI *This,
OUT UINTN *ProcessorNumber
);
Parameters
This
A pointer to the
EDKII_PEI_MP_SERVICES2_PPI
instance.ProcessorNumber
The handle number of AP that is to become the new BSP. The range is from 0 to the total number of logical processors minus 1. The total number of logical processors can be retrieved by
EDKII_PEI_MP_SERVICES2_PPI.GetNumberOfProcessors()
.
Descriptions
This services returns the handle number for the calling processor. This service may be called from the BSP and APs.
This service returns the processor handle number for the calling processor.
The returned value is in the range from 0 to the total
number of logical processors minus 1. The total number of
logical processors can be retrieved with
EDKII_PEI_MP_SERVICES2_PPI.GetNumberOfProcessors()
. This service
may be called from the BSP and APs. If ProcessorNumber
is
NULL, then EFI_INVALID_PARAMETER
is returned. Otherwise,
the current processors handle number is returned in
ProcessorNumber
, and EFI_SUCCESS
is returned.
Status Codes Returned
EFI_SUCCESS |
The current processor handle number was returned in |
EFI_INVALID_PARAMETER |
|
8.3.9.19. EDKII_PEI_MP_SERVICES2_PPI.StartupAllCPUs ()¶
Summary
This service executes a caller provided function on all enabled CPUs.
Prototype
typedef
EFI_STATUS
(EFIAPI *EDKII_PEI_MP_SERVICES_STARTUP_ALL_APS)(
IN EDKII_PEI_MP_SERVICES2_PPI *This,
IN EFI_AP_PROCEDURE Procedure,
IN UINTN TimeoutInMicroSeconds,
IN VOID *ProcedureArgument OPTIONAL
);
Parameters
This
A pointer to the EDKII_PEI_MP_SERVICES2_PPI instance
Procedure
A pointer to the function to be run on enabled APs of the system. See type EFI_AP_PROCEDURE.
TimeoutInMicroseconds
Indicates the time limit in microseconds for APs to return from Procedure, for blocking mode only. Zero means infinity.
If the timeout expires in blocking mode, BSP returns EFI_TIMEOUT..
ProcedureArgument
The parameter passed into Procedure for all APs.
Description
This service executes a caller provided function on all enabled CPUs.
This function is used to dispatch all the enabled CPUs to the function specified by Procedure. If any enabled CPU is busy, then EFI_NOT_READY is returned immediately and Procedure is not started on any AP.
If the timeout specified by TimeoutInMicroseconds expires before all APs return from Procedure, then Procedure on the failed APs is terminated. All enabled APs are always available for further calls to EDKII_PEI_MP_SERVICES2_PPI.StartupAllAPs() and EDKII_PEI_MP_SERVICES2_PPI.StartupThisAP(). If FailedCpuList is not NULL, its content points to the list of processor handle numbers in which Procedure was terminated.
In blocking execution mode, BSP waits until all APs finish or TimeoutInMicroSeconds expires. In non-blocking mode, the function has been dispatched to all enabled CPUs.
Status Codes Returned
EFI_SUCCESS |
In blocking mode, all CPUs have finished before the timeout expired. |
EFI_SUCCESS |
In non-blocking mode, function has been dispatched to all enabled CPUs. |
EFI_DEVICE_ERROR |
Caller processor is AP. |
EFI_NOT_STARTED |
No enabled APs exist in the system. |
EFI_NOT_READY |
Any enabled APs are busy. |
EFI_TIMEOUT |
In blocking mode, the timeout expired before all enabled APs have finished. |
EFI_INVALID_PARAMETER |
Procedure is NULL. |
8.4. Graphics PEIM Interfaces¶
There is one PEI to PEI Interfaces (PPI) that is required to provide graphics functionality in the PEI phase.
The PeiGraphicsPpi
is the PPI produced by the Graphics PEI
Module and provides
interfaces to the platform code to complete the basic
initialization of the graphics
subsystem to enable console output.
8.4.1. Pei Graphics PPI¶
The PeiGraphicsPpi
is the main interface exposed by the
Graphics PEIM to be used by the other firmware modules.
The following sections cover the individual APIs in detail.
GUID
#define EFI_PEI_GRAPHICS_PPI_GUID \
{ 0x6ecd1463, 0x4a4a, 0x461b,
{0xaf, 0x5f, 0x5a, 0x33, 0xe3, 0xb2, 0x16, 0x2b }};
Prototype
struct \_EFI_PEI_GRAPHICS_PPI {
EFI_PEI_GRAPHICS_INIT GraphicsPpiInit;
EFI_PEI_GRAPHICS_GET_MODE GraphicsPpiGetMode;
} EFI_PEI_GRAPHICS_PPI;
8.4.2. GraphicsPpiInit¶
Description
The GraphicsPpiInit initializes the graphics subsystem in phases.
Calling Condition
There are certain conditions to be met before the GraphicsPpiInit can be called; Memory has been initialized.
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_GRAPHICS_INIT) (
IN VOID *GraphicsPolicyPtr;
);
Parameters
GraphicsPolicyPtr
GraphicsPolicyPtr
points to a configuration data block of policy settings required by Graphics PEIM.
Return
EFI_SUCCESS |
The invocation was successful. |
EFI_INVALID_PARAMETER |
The phase parameter is not valid. |
EFI_NOT_ABORTED |
The stages were not called in the proper order |
EFI_NOT_FOUND |
The |
EFI_DEVICE_ERROR |
The initialization failed due to device error |
EFI_NOT_READY |
The previous init stage is still in progress and not ready for the current initialization phase yet. The platform code should call this again sometime later. |
8.4.3. GraphicsPpiGetMode¶
Description
The GraphicsPpiGetMode
returns the mode information
supported by the Graphics PEI Module.
The frame buffer abstracts the video display as an array of pixels. Each pixels location on the video display is defined by its X and Y coordinates. The X coordinate represents a scan line. A scan line is a horizontal line of pixels on the display. The Y coordinate represents a vertical line on the display. The upper left hand corner of the video display is defined as (0, 0) where the notation (X, Y) represents the X and Y coordinate of the pixel. The lower right corner of the video display is represented by (Width -1, Height -1).
A pixel is comprised of a 32-bit quantity. The first three bytes for each pixel represents the intensity for Red, Blue and Green colors. The fourth byte is reserved and must be zero. The byte values for the red, green, and blue components represent the color intensity. This color intensity value range from a minimum intensity of 0 to maximum intensity of 255.
The mode information returned by this PPI is similar to the GOP’s EFI_GRAPHICS_OUTPUT_PROTOCOL_MODE structure.
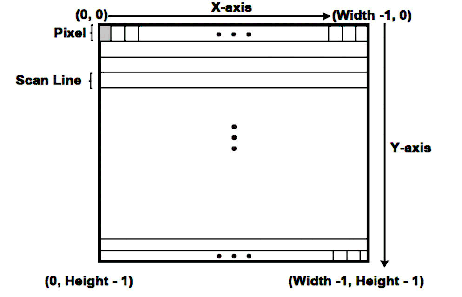
Fig. 8.1 Mode Information Supported By Graphics PEI Module¶
Prototype
typedef
EFI_STATUS
(EFIAPI *EFI_PEI_GRAPHICS_GET_MODE) (
IN OUT EFI_GRAPHICS_OUTPUT_PROTOCOL_MODE *Mode
);
Parameters
Mode
Pointer to
EFI_GRAPHICS_OUTPUT_PROTOCOL_MODE
data. TypeEFI_GRAPHICS_OUTPUT_PROTOCOL_MODE
is defined in theUEFI Specification
and in “Related Definitions” below.
Return
EFI_SUCCESS |
Valid mode information was returned |
EFI_INVALID_PARAMETER |
The |
EFI_NOT_FOUND |
The |
EFI_DEVICE_ERROR |
A hardware error occured trying to retrieve the video mode |
EFI_NOT_READY |
The Graphics Initialization is not compete, and Mode information is not yet available.The platform code should call this again after the Graphics initialization is done. |
8.4.4. EFI PEI Graphics INFO HOB¶
8.4.4.1. EFI_PEI_GRAPHICS_INFO_HOB¶
#define EFI_PEI_GRAPHICS_INFO_HOB_GUID \
{ 0x39f62cce, 0x6825, 0x4669,\
{ 0xbb, 0x56, 0x54, 0x1a, 0xba, 0x75, 0x3a, 0x07 }}
typedef struct {
EFI_PHYSICAL_ADDRESS FrameBufferBase;
UINT32 FrameBufferSize;
EFI_GRAPHICS_OUTPUT_MODE_INFORMATION GraphicsMode;
} EFI_PEI_GRAPHICS_INFO_HOB;
8.4.4.2. EFI_PEI_GRAPHICS_DEVICE_INFO_HOB¶
#define EFI_PEI_GRAPHICS_DEVICE_INFO_HOB_GUID \
{ 0xe5cb2ac9, 0xd35d, 0x4430,\
{ 0x93, 0x6e, 0x1d, 0xe3, 0x32, 0x47, 0x8d, 0xe7 }}
typedef struct {
UINT16 VendorId
UINT16 DeviceId
UINT16 SubsystemVendorId
UINT16 SubsystemId;
UINT8 RevisionId;
UINT8 BarIndex;
} EFI_PEI_GRAPHICS_DEVICE_INFO_HOB;
When graphics capability is included in PEI, it may optionally provide a splash screen capability as well.
When graphics capability is included in PEI, it produces a
EFI_PEI_GRAPHICS_INFO_HOB
which provides information about
the graphics mode and the framebuffer, and may optionally
produce a EFI_PEI_GRAPHICS_DEVICE_INFO_HOB which provides
information about the graphics device characteristics. The
EFI_GRAPHICS_OUTPUT_MODE_INFORMATION
structure is defined in
the UEFI specification. This information can be used by the
HOB-consumer phase, such as DXE, to provide display support of
its own, or elide the need to do graphics initialization again
in the UEFI GOP driver, for example.
It is to be noted that the PEI phase may program a temporary
framebuffer address to complete its initialization and the
framebuffer address at the time of building the
EFI_PEI_GRAPHICS_INFO_HOB
will reflect the current
assignment. The post-PEI phase consuming this HOB should be
aware that a generic PCI enumeration logic could reprogram the
temporary resources assigned by the PEI phase and it is the
responsibility of the post-PEI phase to update its internal
data structures with the new framebuffer address after the
enumeration is complete.
The EFI_PEI_GRAPHICS_DEVICE_INFO_HOB is optional. When it exists, the DXE module which provides display support uses the VendorId, DeviceId, RevisionId, SubsystemVendorId, and SubsystemDeviceId in the HOB to match the graphics device. It’s useful when system has multiple graphics devices and the DXE module cannot know which one to manage without the information provided by this HOB. If VendorId, DeviceId, SubsystemVendorId or SubsystemDeviceId is set to MAX_UINT16, or RevisionId is set to MAX_UINT8, that field will be ignored. The ID values that are assigned to other values will be used to identify the graphics device. The BarIndex tells DXE module which PCI MMIO BAR is used to hold the frame buffer. BAR 0 is used if the BarIndex is set to MAX_UINT8 or the HOB doesn’t exist.